Hello everyone!
I am entirely new to trading, MQL5, or coding in general. Trying to learn something new during the lockdown days so please excuse my ignorance.
I am basically trying to code an EA that will open or close positions based on the profit of my most recent open position. I have found that I should probably use PositionGetDouble( POSITION_PROFIT ) but I am quite confused as to how I should arrange my cache to include only the most recent open position and adjust automatically as the EA opens and closes new positions.
I would really appreciate any and all help.
Thank you very much in advance!
Sample code.
Uses trade classes
Class / Group |
Description |
Class for working with open position properties |
|
Class for working with history deal properties |
|
Class for trade operations execution |
The EA does not work with the trading history (it is assumed that the EA works all the time, the EA will not turn off, you will not switch the timeframe on the chart). How the adviser catches the moment of closing a position - it catches the closing of a position in OnTradeTransaction : waiting for the transaction TRADE_TRANSACTION_DEAL_ADD - adding a deal to history .
As soon as the deal "TRADE_TRANSACTION_DEAL_ADD - adding a deal to history" is caught, the adviser displays a comment on the chart. ATTENTION: the transaction is searched by the current symbol and BY THE PRESET Magic Number
//+------------------------------------------------------------------+ //| Simple EA Based Latest Position.mq5 | //| Copyright © 2020, Vladimir Karputov | //+------------------------------------------------------------------+ #property copyright "Copyright © 2020, Vladimir Karputov" #property version "1.000" //--- #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> #include <Trade\DealInfo.mqh> //--- CPositionInfo m_position; // object of CPositionInfo class CTrade m_trade; // object of CTrade class CDealInfo m_deal; // object of CDealInfo class //--- input parameters input ulong InpDeviation = 10; // Deviation, in points (1.00045-1.00055=10 points) input ulong InpMagic = 200; // Magic number //--- ENUM_POSITION_TYPE m_last_position_type=WRONG_VALUE; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- m_trade.SetExpertMagicNumber(InpMagic); m_trade.SetMarginMode(); m_trade.SetTypeFillingBySymbol(Symbol()); m_trade.SetDeviationInPoints(InpDeviation); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //--- get transaction type as enumeration value ENUM_TRADE_TRANSACTION_TYPE type=trans.type; //--- if transaction is result of addition of the transaction in history if(type==TRADE_TRANSACTION_DEAL_ADD) { if(HistoryDealSelect(trans.deal)) m_deal.Ticket(trans.deal); else return; if(m_deal.Symbol()==Symbol() && m_deal.Magic()==InpMagic) { if(m_deal.Entry()==DEAL_ENTRY_OUT) { if(m_deal.DealType()==DEAL_TYPE_BUY) { m_last_position_type=POSITION_TYPE_SELL; Comment("The position SELL has been closed"); } if(m_deal.DealType()==DEAL_TYPE_SELL) { m_last_position_type=POSITION_TYPE_BUY; Comment("The position BUY has been closed"); } } } } } //+------------------------------------------------------------------+
Code:
//+------------------------------------------------------------------+ //| Simple EA Based Latest Position.mq5 | //| Copyright © 2020, Vladimir Karputov | //+------------------------------------------------------------------+ #property copyright "Copyright © 2020, Vladimir Karputov" #property version "1.000" //--- #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> #include <Trade\DealInfo.mqh> //--- CPositionInfo m_position; // object of CPositionInfo class CTrade m_trade; // object of CTrade class CDealInfo m_deal; // object of CDealInfo class //--- input parameters input ulong InpDeviation = 10; // Deviation, in points (1.00045-1.00055=10 points) input ulong InpMagic = 200; // Magic number //--- ENUM_POSITION_TYPE m_last_position_type=WRONG_VALUE; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- m_trade.SetExpertMagicNumber(InpMagic); m_trade.SetMarginMode(); m_trade.SetTypeFillingBySymbol(Symbol()); m_trade.SetDeviationInPoints(InpDeviation); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //--- get transaction type as enumeration value ENUM_TRADE_TRANSACTION_TYPE type=trans.type; //--- if transaction is result of addition of the transaction in history if(type==TRADE_TRANSACTION_DEAL_ADD) { if(HistoryDealSelect(trans.deal)) m_deal.Ticket(trans.deal); else return; if(m_deal.Symbol()==Symbol() && m_deal.Magic()==InpMagic) { if(m_deal.Entry()==DEAL_ENTRY_OUT) { if(m_deal.DealType()==DEAL_TYPE_BUY) { m_last_position_type=POSITION_TYPE_SELL; Comment("The position SELL has been closed"); } if(m_deal.DealType()==DEAL_TYPE_SELL) { m_last_position_type=POSITION_TYPE_BUY; Comment("The position BUY has been closed"); } } } } } //+------------------------------------------------------------------+
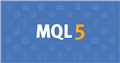
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone!
I am entirely new to trading, MQL5, or coding in general. Trying to learn something new during the lockdown days so please excuse my ignorance.
I am basically trying to code an EA that will open or close positions based on the profit of my most recent open position. I have found that I should probably use PositionGetDouble(POSITION_PROFIT) but I am quite confused as to how I should arrange my cache to include only the most recent open position and adjust automatically as the EA opens and closes new positions.
I would really appreciate any and all help.
Thank you very much in advance!