- MetaEditor - Professional editor of trading applications
- MQL5 Wizard: Development of trading robots for MetaTrader 5
- MetaTrader 5 for your employees
There is nothing complicated in MQL5. The main thing you should do: uproot the word “order” from your head, and use the new word “position” and “pending order” instead.
Position is: BUY and SELL.
A pending order is: Buy stop, Buy limit, Sell stop, Sell limit ...
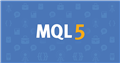
- www.mql5.com
As I promised - here is the code. Here (just in case) a verification of the success of the modification is introduced. This check increased the code, but in case of an error it will be possible to see.
//+------------------------------------------------------------------+ //| ChartPriceOnDropped Modification.mq5 | //| Copyright © 2019, Vladimir Karputov | //| http://wmua.ru/slesar/ | //+------------------------------------------------------------------+ #property copyright "Copyright © 2019, Vladimir Karputov" #property link "http://wmua.ru/slesar/" #property version "1.000" #property script_show_inputs //--- #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> #include <Trade\OrderInfo.mqh> //--- CPositionInfo m_position; // object of CPositionInfo class CTrade m_trade; // object of CTrade class CSymbolInfo m_symbol; // object of CSymbolInfo class COrderInfo m_order; // object of COrderInfo class //--- input ulong InpDeviation = 10; // Deviation, in points (1.00045-1.00055=10 points) input bool InpPrintLog = false; // Print log input ulong InpMagic = 456; // Magic number //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- if(!m_symbol.Name(Symbol())) // sets symbol name { Print(__FILE__," ",__FUNCTION__,", ERROR: CSymbolInfo.Name"); return; } RefreshRates(); //--- m_trade.SetExpertMagicNumber(InpMagic); m_trade.SetMarginMode(); m_trade.SetTypeFillingBySymbol(m_symbol.Name()); m_trade.SetDeviationInPoints(InpDeviation); //--- double price_on_dropped=ChartPriceOnDropped(); for(int i=PositionsTotal()-1; i>=0; i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.Symbol()==m_symbol.Name()) { double price_current = m_position.PriceCurrent(); double stop_loss = m_position.StopLoss(); //--- if(m_position.PositionType()==POSITION_TYPE_BUY) { if(price_on_dropped>price_current) { if(!m_trade.PositionModify(m_position.Ticket(), stop_loss, m_symbol.NormalizePrice(price_on_dropped))) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify BUY ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } else { if(price_on_dropped<price_current) { if(!m_trade.PositionModify(m_position.Ticket(), stop_loss, m_symbol.NormalizePrice(price_on_dropped))) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify SELL ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } } for(int i=OrdersTotal()-1; i>=0; i--) // returns the number of current orders if(m_order.SelectByIndex(i)) // selects the pending order by index for further access to its properties if(m_order.Symbol()==m_symbol.Name()) { double price_current = m_order.PriceCurrent(); double price_open = m_order.PriceOpen(); double stop_loss = m_order.StopLoss(); ENUM_ORDER_TYPE_TIME type_time=m_order.TypeTime(); datetime expiration = m_order.TimeExpiration(); //--- if(m_order.OrderType()==ORDER_TYPE_BUY_LIMIT) { if(price_on_dropped>price_open) { if(!m_trade.OrderModify(m_order.Ticket(),price_open, stop_loss, m_symbol.NormalizePrice(price_on_dropped), type_time,expiration)) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify Buy limit ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } else if(m_order.OrderType()==ORDER_TYPE_SELL_LIMIT) { if(price_on_dropped<price_open) { if(!m_trade.OrderModify(m_order.Ticket(),price_open, stop_loss, m_symbol.NormalizePrice(price_on_dropped), type_time,expiration)) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify Sell limit ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } else if(m_order.OrderType()==ORDER_TYPE_BUY_STOP) { if(price_on_dropped>price_open) { if(!m_trade.OrderModify(m_order.Ticket(),price_open, stop_loss, m_symbol.NormalizePrice(price_on_dropped), type_time,expiration)) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify Buy stop ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } else if(m_order.OrderType()==ORDER_TYPE_SELL_STOP) { if(price_on_dropped<price_open) { if(!m_trade.OrderModify(m_order.Ticket(),price_open, stop_loss, m_symbol.NormalizePrice(price_on_dropped), type_time,expiration)) if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Modify Sell stop ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } } } //+------------------------------------------------------------------+ //| Refreshes the symbol quotes data | //+------------------------------------------------------------------+ bool RefreshRates() { //--- refresh rates if(!m_symbol.RefreshRates()) { if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","RefreshRates error"); return(false); } //--- protection against the return value of "zero" if(m_symbol.Ask()==0 || m_symbol.Bid()==0) { if(InpPrintLog) Print(__FILE__," ",__FUNCTION__,", ERROR: ","Ask == 0.0 OR Bid == 0.0"); return(false); } //--- return(true); } //+------------------------------------------------------------------+
As I promised - here is the code. Here (just in case) a verification of the success of the modification is introduced. This check increased the code, but in case of an error it will be possible to see.
I CAN'T THANK YOU ENOUGH, YOU SAVED ME.. i was so stressed, i couldn't think of anything else, this is the best day of my life.. i am trying to learn Advance level of MQL5, i hope i succeed, you really motivated me, thank you sir Vladimir Karputov :)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use