CPositionInfo: How does SelectByMagic work if there are multiple positions with the same magic number?
- Questions from Beginners MQL5 MT5 MetaTrader 5
- Detect stop trigger in OnTradeTransaction backtest
- Errors, bugs, questions
The title says it all. The documentation of CPositionInfo is not complete at this point. Is then simply the first applicable position selected? If so, how is "first" defined? The first in time? I would be very grateful for answers with a reference to the source.
I suppose this function is useful for a netting account or if you know you have only 1 position for a given symbol.
//+------------------------------------------------------------------+ //| Access functions PositionSelect(...) | //+------------------------------------------------------------------+ bool CPositionInfo::SelectByMagic(const string symbol,const ulong magic) { bool res=false; uint total=PositionsTotal(); //--- for(uint i=0; i<total; i++) { string position_symbol=PositionGetSymbol(i); if(position_symbol==symbol && magic==PositionGetInteger(POSITION_MAGIC)) { res=true; break; } } //--- return(res); }
It selects the first position encountered.
I suppose this function is useful for a netting account or if you know you have only 1 position for a given symbol.
It selects the first position encountered.
Alain Verleyen #:
I suppose this function is useful for a netting account or if you know you have only 1 position for a given symbol.
It selects the first position encountered.
Hi all,
Resurrecting this thread as I too found myself bumping my head into the apparent "lack of logic" in CPositionInfo::SelectByMagic() -- this is something I came across in the course of studying (and playing with) the Strategy Modules of the Standard Library, something I embarked on a few weeks ago and not regretting yet ;-) despite the numerous challenges (see my other post, if interested).
Searching across the whole MQL5 directory and found this method is only ever used by CExpert::SelectPosition(), so not heavily used by any stretch of the imagination (unsurprisingly, I must say).
$ grep -r SelectByMagic MQL5/ MQL5/Include/Expert/Expert.mqh: res=m_position.SelectByMagic(m_symbol.Name(),m_magic); MQL5/Include/Trade/PositionInfo.mqh: bool SelectByMagic(const string symbol,const ulong magic); MQL5/Include/Trade/PositionInfo.mqh: bool CPositionInfo::SelectByMagic(const string symbol,const ulong magic)
To @Alain Verleyen's point above, yes, netting & hedging accounts come into play here and (supposedly) selecting the first position encountered in the latter case... This was a sticking point for my requirements for managing multiple positions.
//+------------------------------------------------------------------+ //| Position select depending on netting or hedging | //+------------------------------------------------------------------+ bool CExpert::SelectPosition(void) { bool res=false; //--- if(IsHedging()) res=m_position.SelectByMagic(m_symbol.Name(),m_magic); else res=m_position.Select(m_symbol.Name()); //--- return(res); }
I may be missing something, but in my study of the CExpert* family of classes I found out quite a few limitations including the ability to manage multiple positions within that OOP framework out-of-the-box. My guess is that probably because this part of the Standard Library is mostly used by the MQL Wizard and not a widely adopted by the MQL developer community at large, one cannot expect too much here... Dare I say, MetaQuotes are not actively developing & supporting this?
On closer inspection, CExpert appears to include some preliminay / half-baked / unfinished support to allow for multiple trades like having a m_max_orders member declared but never used). At any rate, I decided to roll a custom version of the class, i.e. CMyExpert, to better address my requirements for modularity/flexibility and also modernise it to use the OnTradeTransaction() event handler instead of the older, parameter-less OnTrade() function.
Not to derail this post further off-topic, let me get back to ploughing on with this work... I'd be keen read others' views around these topics though.
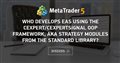
- 2022.06.03
- www.mql5.com
When MT5/MQL5 first came out it ONLY supported the "Netting" modal, and everything was geared towards it, even the Standard Library.
Only later (I am not sure when, but I believe a year or two), did MetaQuotes finally decide to listen to the outcry by customers and brokers alike, an add the "Hedging" mode.
This is the reason there are remnants of functions and class methods that only apply to the "Netting" modal and do not work with "Hedging" mode.
When you want to manage a group of positions, like those that have a common magic number, you would need a position group manager.
Obviously, CPositionInfo , as it's name suggests, is for managing one positoon only.
When you want to manage a group of positions, like those that have a common magic number, you would need a position group manager.
From Documentation:
CPositionInfo is a class for easy access to the open position properties.
BUT: You yourself determine which position you need to choose!
Standard position cycle example (works for both netting and hedging) - here the position is selected through its index:
//+------------------------------------------------------------------+ //| Calculate all positions | //+------------------------------------------------------------------+ int CalculateAllPositions(void) { int total=0; for(int i=PositionsTotal()-1; i>=0; i--) if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties if(m_position.Symbol()==m_symbol.Name() && m_position.Magic()==InpMagic) total++; //--- return(total); }
From Documentation:
CPositionInfo is a class for easy access to the open position properties.
BUT: You yourself determine which position you need to choose!
Standard position cycle example (works for both netting and hedging) - here the position is selected through its index:
Yes, I know. I just meant that if you want to select multiple positions, like 10 positions by SelectByMagic(123456) for instance, you need a group positions class.
Thank you all, for your insights -- it helps get a better understanding of the historical context and usage patterns...
Amir Yacoby #:
Yes, I know. I just meant that if you want to select multiple positions, like 10 positions by SelectByMagic(123456) for instance, you need a group positions class.
@Amir Yacoby -- have you already coded such a positions group class, or how would you go about doing that? I'm still busy with some other parts of the code, before coming back to work on designing a solution for multi-position management... Maybe yours can serve as a guide or inspiration ;-)
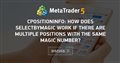
- 2019.09.19
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use