You should read the documentation, specially when you are "new at programming in mql5.
You can't call iMA (or a function to get an indicator handle in general) and in the next statement use CopyBuffer(), the indicator is not ready
yet. Use iMA in OnInit() and CopyBuffer() in Ontick(), as shown in the documentation example.
You should read the documentation, specially when you are "new at programming in mql5.
You can't call iMA (or a function to get an indicator handle in general) and in the next statement use CopyBuffer(), the indicator is not
ready yet. Use iMA in OnInit() and CopyBuffer() in Ontick(), as shown in the documentation example.
i have the same problem! your reply is so helpful ! thanks a lot.
You should read the documentation, specially when you are "new at programming in mql5.
You can't call iMA (or a function to get an indicator handle in general) and in the next statement use CopyBuffer(), the indicator is not ready yet. Use iMA in OnInit() and CopyBuffer() in Ontick(), as shown in the documentation example.
Can You Help Me, I Have Same Error In Mq5
Creating an iMA indicator handle, getting indicator values
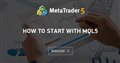
- 2020.03.05
- www.mql5.com
You should read the documentation, specially when you are "new at programming in mql5.
You can't call iMA (or a function to get an indicator handle in general) and in the next statement use CopyBuffer(), the indicator is not ready yet. Use iMA in OnInit() and CopyBuffer() in Ontick(), as shown in the documentation example.
But next code working fine at my end
double getIndicator(int v) { int handle = iCustom(curSymbol,Indicator_Period,"Market\\Entry Points Pro for MT5"); //,1000,true); // ,false,false,500,false,"09:00","23:59",false //,30,255,3329330,14772545,9,true,true,true,"alert2.wav" double myArray[]; int copy=CopyBuffer(handle,v,0,1,myArray); return (double)myArray[0]; }
MQL5 style, the indicato
This is very bad. Because if I am monitoring several symbols, like, 20 symbols, I am making a function like:
double arrayADXmainline[]; double arrayPlusADXline[]; double arrayMinusADXline[]; double ADX(string symbol, ENUM_TIMEFRAMES timeframe, int comprimento, int modo, int posicao) //ADX(_Symbol,_Period,4,4,0) { int adxHandle=iADX(symbol,timeframe,8); ArrayResize(arrayADXmainline,comprimento); ArrayResize(arrayPlusADXline,comprimento); ArrayResize(arrayMinusADXline,comprimento); ArraySetAsSeries(arrayADXmainline,true); ArraySetAsSeries(arrayPlusADXline,true); ArraySetAsSeries(arrayMinusADXline,true); CopyBuffer(adxHandle,MAIN_LINE,0,comprimento,arrayADXmainline); CopyBuffer(adxHandle,PLUSDI_LINE,0,comprimento,arrayPlusADXline); CopyBuffer(adxHandle,MINUSDI_LINE,0,comprimento,arrayMinusADXline); int sign=1; double variavelRetorno=0; if(modo==1) { if(arrayPlusADXline[posicao]<arrayMinusADXline[posicao]) { sign=-1; return sign; } else { sign=1; return sign; } }//if modo == 1 if(modo==2) { return arrayPlusADXline[posicao]; }//if modo == 2 if(modo==3) { return arrayMinusADXline[posicao]; }//if modo == 3 if(modo==4) { Print("TESTE1"); variavelRetorno = arrayPlusADXline[0]-arrayMinusADXline[0]; Print("TESTE"); return variavelRetorno; }//if modo == 4 if(modo==5) { if(arrayPlusADXline[1]-arrayMinusADXline[1]>0) if(arrayPlusADXline[2]-arrayMinusADXline[2]<0) return 1; if(arrayPlusADXline[1]-arrayMinusADXline[1]<0) if(arrayPlusADXline[2]-arrayMinusADXline[2]>0) return 1; return 0; }//if modo == 5 return 0; } //----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
How I am supposed to split the code between onInit() and onTick() function ?
By the way, because what I want is just to monitor, I am not even using ontick(). I call the funcion I did in ontimer() every 60 seconds.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone,
I am completely new at programming in mql5 and unfortunately I have some problems to build my code based on a simple moving average.
I am basically trying to :
When I compile my code, there is no error. But when I backtest it the following error appear in the journal :
"Cannot load indicator 'Moving Average' [4002]"
Could someone tell me why I have this "input"?
Thank you a lot for your time