Hello friend,
Try this:
//+------------------------------------------------------------------+ //| Heiken Ashi.mq4 | //| Copyright 2006-2014, MetaQuotes Software Corp. | //| http://www.mql4.com | //+------------------------------------------------------------------+ #property copyright "2006-2014, MetaQuotes Software Corp." #property link "http://www.mql4.com" #property description "We recommend next chart settings (press F8 or select menu 'Charts'->'Properties...'):" #property description " - on 'Color' Tab select 'Black' for 'Line Graph'" #property description " - on 'Common' Tab disable 'Chart on Foreground' checkbox and select 'Line Chart' radiobutton" #property strict #property indicator_chart_window #property indicator_buffers 4 #property indicator_color1 Red #property indicator_color2 White #property indicator_color3 Blue #property indicator_color4 Green #property indicator_width1 1 #property indicator_width2 1 #property indicator_width3 3 #property indicator_width4 3 //--- input color ExtColor1 = Red; // Shadow of bear candlestick input color ExtColor2 = White; // Shadow of bull candlestick input color ExtColor3 = Blue; // Bear candlestick body input color ExtColor4 = Green; // Bull candlestick body //--- buffers double ExtLowHighBuffer[]; double ExtHighLowBuffer[]; double ExtOpenBuffer[]; double ExtCloseBuffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //|------------------------------------------------------------------| void OnInit(void) { IndicatorShortName("Heiken Ashi"); IndicatorDigits(Digits); //--- indicator lines SetIndexStyle(0,DRAW_HISTOGRAM,0,1,ExtColor1); SetIndexBuffer(0,ExtLowHighBuffer); SetIndexStyle(1,DRAW_HISTOGRAM,0,1,ExtColor2); SetIndexBuffer(1,ExtHighLowBuffer); SetIndexStyle(2,DRAW_HISTOGRAM,0,3,ExtColor3); SetIndexBuffer(2,ExtOpenBuffer); SetIndexStyle(3,DRAW_HISTOGRAM,0,3,ExtColor4); SetIndexBuffer(3,ExtCloseBuffer); //--- SetIndexLabel(0,"Low/High"); SetIndexLabel(1,"High/Low"); SetIndexLabel(2,"Open"); SetIndexLabel(3,"Close"); SetIndexDrawBegin(0,10); SetIndexDrawBegin(1,10); SetIndexDrawBegin(2,10); SetIndexDrawBegin(3,10); //--- indicator buffers mapping SetIndexBuffer(0,ExtLowHighBuffer); SetIndexBuffer(1,ExtHighLowBuffer); SetIndexBuffer(2,ExtOpenBuffer); SetIndexBuffer(3,ExtCloseBuffer); //--- initialization done } //+------------------------------------------------------------------+ //| Heiken Ashi | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int i,pos; double haOpen,haHigh,haLow,haClose; //--- if(rates_total<=10) return(0); //--- counting from 0 to rates_total ArraySetAsSeries(ExtLowHighBuffer,false); ArraySetAsSeries(ExtHighLowBuffer,false); ArraySetAsSeries(ExtOpenBuffer,false); ArraySetAsSeries(ExtCloseBuffer,false); ArraySetAsSeries(open,false); ArraySetAsSeries(high,false); ArraySetAsSeries(low,false); ArraySetAsSeries(close,false); //--- preliminary calculation if(prev_calculated>1) pos=prev_calculated-1; else { //--- set first candle if(open[0]<close[0]) { ExtLowHighBuffer[0]=low[0]; ExtHighLowBuffer[0]=high[0]; } else { ExtLowHighBuffer[0]=high[0]; ExtHighLowBuffer[0]=low[0]; } ExtOpenBuffer[0]=open[0]; ExtCloseBuffer[0]=close[0]; //--- pos=1; } //--- main loop of calculations for(i=pos; i<rates_total; i++) { haOpen=(ExtOpenBuffer[i-1]+ExtCloseBuffer[i-1])/2; haClose=(open[i]+high[i]+low[i]+close[i])/4; haHigh=MathMax(high[i],MathMax(haOpen,haClose)); haLow=MathMin(low[i],MathMin(haOpen,haClose)); if(haOpen<haClose) { ExtLowHighBuffer[i]=haLow; ExtHighLowBuffer[i]=haHigh; } else { ExtLowHighBuffer[i]=haHigh; ExtHighLowBuffer[i]=haLow; } ExtOpenBuffer[i]=haOpen; ExtCloseBuffer[i]=haClose; } //--- done return(rates_total); } //+------------------------------------------------------------------+
//+------------------------------------------------------------------+ //| Heiken Ashi Smoothed.mq4 | //| | //| mod by Raff | //+------------------------------------------------------------------+ #property copyright "Copyright © 2006, Forex-TSD.com " #property link "http://www.forex-tsd.com/" #property strict //---- #property indicator_chart_window #property indicator_buffers 8 #property indicator_color1 Red #property indicator_color2 White #property indicator_color3 Blue #property indicator_color4 Green //---- parameters extern int MaMetod =2; extern int MaPeriod=6; extern int MaMetod2 =3; extern int MaPeriod2=2; //---- buffers double ExtMapBuffer1[]; double ExtMapBuffer2[]; double ExtMapBuffer3[]; double ExtMapBuffer4[]; double ExtMapBuffer5[]; double ExtMapBuffer6[]; double ExtMapBuffer7[]; double ExtMapBuffer8[]; //---- int ExtCountedBars=0; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //|------------------------------------------------------------------| int init() { //---- indicators IndicatorBuffers(8); SetIndexStyle(0,DRAW_HISTOGRAM,0,1,Red); SetIndexBuffer(0,ExtMapBuffer1); SetIndexStyle(1,DRAW_HISTOGRAM,0,1,White); SetIndexBuffer(1,ExtMapBuffer2); SetIndexStyle(2,DRAW_HISTOGRAM,0,3,Blue); SetIndexBuffer(2,ExtMapBuffer3); SetIndexStyle(3,DRAW_HISTOGRAM,0,3,Green); SetIndexBuffer(3,ExtMapBuffer4); //---- SetIndexDrawBegin(0,5); //---- indicator buffers mapping SetIndexBuffer(0,ExtMapBuffer1); SetIndexBuffer(1,ExtMapBuffer2); SetIndexBuffer(2,ExtMapBuffer3); SetIndexBuffer(3,ExtMapBuffer4); SetIndexBuffer(4,ExtMapBuffer5); SetIndexBuffer(5,ExtMapBuffer6); SetIndexBuffer(6,ExtMapBuffer7); SetIndexBuffer(7,ExtMapBuffer8); //---- initialization done return(0); } //+------------------------------------------------------------------+ //| Custor indicator deinitialization function | //+------------------------------------------------------------------+ int deinit() { //---- TODO: add your code here //---- return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int start() { double maOpen,maClose,maLow,maHigh; double haOpen,haHigh,haLow,haClose; if(Bars<=10) return(0); int counted_bars=IndicatorCounted(); if(counted_bars<0) return(-1); if(counted_bars>0) counted_bars--; int limit=Bars-counted_bars; if(counted_bars==0) limit-=1+MathMax(1,MathMax(MaPeriod,MaPeriod2)); int pos=limit; while(pos>=0) { maOpen=iMA(NULL,0,MaPeriod,0,MODE_SMA,PRICE_OPEN,pos); maClose=iMA(NULL,0,MaPeriod,0,MODE_SMA,PRICE_CLOSE,pos); maLow=iMA(NULL,0,MaPeriod,0,MODE_SMA,PRICE_LOW,pos); maHigh=iMA(NULL,0,MaPeriod,0,MODE_SMA,PRICE_HIGH,pos); //---- haOpen=(ExtMapBuffer5[pos+1]+ExtMapBuffer6[pos+1])/2; haClose=(maOpen+maHigh+maLow+maClose)/4; haHigh=MathMax(maHigh,MathMax(haOpen,haClose)); haLow=MathMin(maLow,MathMin(haOpen,haClose)); if(haOpen<haClose) { ExtMapBuffer7[pos]=haLow; ExtMapBuffer8[pos]=haHigh; } else { ExtMapBuffer7[pos]=haHigh; ExtMapBuffer8[pos]=haLow; } ExtMapBuffer5[pos]=haOpen; ExtMapBuffer6[pos]=haClose; pos--; } int i; for(i=0; i<limit; i++) ExtMapBuffer1[i]=iMAOnArray(ExtMapBuffer7,0,MaPeriod2,0,MaMetod2,i); for(i=0; i<limit; i++) ExtMapBuffer2[i]=iMAOnArray(ExtMapBuffer8,0,MaPeriod2,0,MaMetod2,i); for(i=0; i<limit; i++) ExtMapBuffer3[i]=iMAOnArray(ExtMapBuffer5,0,MaPeriod2,0,MaMetod2,i); for(i=0; i<limit; i++) ExtMapBuffer4[i]=iMAOnArray(ExtMapBuffer6,0,MaPeriod2,0,MaMetod2,i); //---- return(0); } //+------------------------------------------------------------------+
Change everything to what you want, save a template as default.
Change everything to what you want, save a template as default.
int init() { //---- indicators IndicatorBuffers(8); SetIndexStyle(0,DRAW_HISTOGRAM); SetIndexBuffer(0, ExtMapBuffer1); SetIndexStyle(1,DRAW_HISTOGRAM); SetIndexBuffer(1, ExtMapBuffer2); SetIndexStyle(2,DRAW_HISTOGRAM); SetIndexBuffer(2, ExtMapBuffer3); SetIndexStyle(3,DRAW_HISTOGRAM); SetIndexBuffer(3, ExtMapBuffer4); //---- SetIndexDrawBegin(0,5); //---- indicator buffers mapping SetIndexBuffer(4,ExtMapBuffer5); SetIndexBuffer(5,ExtMapBuffer6); SetIndexBuffer(6,ExtMapBuffer7); SetIndexBuffer(7,ExtMapBuffer8); //---- initialization done return(0); }
#property indicator_width1 3 #property indicator_width2 3 #property indicator_width3 1 #property indicator_width4 1
Add above 4 lines and delete style, width and color from "SetIndexStyle".
Delete 4 "SetIndexBuffer" because no need to declare 2 times.
Do not double post.
I have deleted your duplicated topic and moved the replies here.
Hello friends,
I have been trying to help the OP as much as possible.
#property indicator_chart_window #property indicator_buffers 4 #property indicator_color1 Red #property indicator_color2 Lime #property indicator_color3 Red #property indicator_color4 Lime //---- parameters extern int MaMetod =2; extern int MaPeriod=6; extern int MaMetod2 =3; extern int MaPeriod2=2; //---- buffers double ExtMapBuffer1[]; double ExtMapBuffer2[]; double ExtMapBuffer3[]; double ExtMapBuffer4[]; double ExtMapBuffer5[]; double ExtMapBuffer6[]; double ExtMapBuffer7[]; double ExtMapBuffer8[];
You don't listen.
Should have caused an error.
maOpen=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_OPEN,pos); maClose=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_CLOSE,pos); maLow=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_LOW,pos); maHigh=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_HIGH,pos);
Should have caused an error.
hi everyone thanks for input.
im not sure what iv done and pretty sure ive screwed things up the indicator is now staying at the colours I want an so are the line thickness. the only remaining issue is that the candles will not stay on the foreground.
the only error that comes up is - improper enumerator cannot be used which is repeated 4 times although the indicator is working ok. also might have screwwed up the deleat buffer part
heres the code as it is right now
//+------------------------------------------------------------------+
//| Heiken Ashi Smoothed.mq4 |
//| |
//| mod by Raff |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2006, Forex-TSD.com "
#property link "http://www.forex-tsd.com/"
//----
#property indicator_chart_window
#property indicator_buffers 4
#property indicator_color1 Red
#property indicator_color2 Lime
#property indicator_color3 Red
#property indicator_color4 Lime
//---- parameters
extern int MaMetod =2;
extern int MaPeriod=6;
extern int MaMetod2 =3;
extern int MaPeriod2=2;
//---- buffers
double ExtMapBuffer1[];
double ExtMapBuffer2[];
double ExtMapBuffer3[];
double ExtMapBuffer4[];
double ExtMapBuffer5[];
double ExtMapBuffer6[];
double ExtMapBuffer7[];
double ExtMapBuffer8[];
//----
int ExtCountedBars=0;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//|------------------------------------------------------------------|
int init()
{
//---- indicators
IndicatorBuffers(8);
SetIndexStyle(0,DRAW_HISTOGRAM);
SetIndexBuffer(0, ExtMapBuffer1);
SetIndexStyle(1,DRAW_HISTOGRAM);
SetIndexBuffer(1, ExtMapBuffer2);
SetIndexStyle(2,DRAW_HISTOGRAM);
SetIndexBuffer(2, ExtMapBuffer3);
SetIndexStyle(3,DRAW_HISTOGRAM);
SetIndexBuffer(3, ExtMapBuffer4);
//----
SetIndexDrawBegin(0,5);
//---- indicator buffers mapping
SetIndexBuffer(4,ExtMapBuffer5);
SetIndexBuffer(5,ExtMapBuffer6);
SetIndexBuffer(6,ExtMapBuffer7);
SetIndexBuffer(7,ExtMapBuffer8);
//---- initialization done
return(0);
}
//+------------------------------------------------------------------+
//| Custor indicator deinitialization function |
//+------------------------------------------------------------------+
int deinit()
{
//---- TODO: add your code here
//----
return(0);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
double maOpen, maClose, maLow, maHigh;
double haOpen, haHigh, haLow, haClose;
if(Bars<=10) return(0);
ExtCountedBars=IndicatorCounted();
//---- check for possible errors
if (ExtCountedBars<0) return(-1);
//---- last counted bar will be recounted
if (ExtCountedBars>0) ExtCountedBars--;
int pos=Bars-ExtCountedBars-1;
while(pos>=0)
{
maOpen=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_OPEN,pos);
maClose=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_CLOSE,pos);
maLow=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_LOW,pos);
maHigh=iMA(NULL,0,MaPeriod,0,MaMetod,MODE_HIGH,pos);
//----
haOpen=(ExtMapBuffer5[pos+1]+ExtMapBuffer6[pos+1])/2;
haClose=(maOpen+maHigh+maLow+maClose)/4;
haHigh=MathMax(maHigh, MathMax(haOpen, haClose));
haLow=MathMin(maLow, MathMin(haOpen, haClose));
if (haOpen<haClose)
{
ExtMapBuffer7[pos]=haLow;
ExtMapBuffer8[pos]=haHigh;
}
else
{
ExtMapBuffer7[pos]=haHigh;
ExtMapBuffer8[pos]=haLow;
}
ExtMapBuffer5[pos]=haOpen;
ExtMapBuffer6[pos]=haClose;
pos--;
}
int i;
for(i=0; i<Bars; i++) ExtMapBuffer1[i]=iMAOnArray(ExtMapBuffer7,Bars,MaPeriod2,0,MaMetod2,i);
for(i=0; i<Bars; i++) ExtMapBuffer2[i]=iMAOnArray(ExtMapBuffer8,Bars,MaPeriod2,0,MaMetod2,i);
for(i=0; i<Bars; i++) ExtMapBuffer3[i]=iMAOnArray(ExtMapBuffer5,Bars,MaPeriod2,0,MaMetod2,i);
for(i=0; i<Bars; i++) ExtMapBuffer4[i]=iMAOnArray(ExtMapBuffer6,Bars,MaPeriod2,0,MaMetod2,i);
//----
return(0);
}
//+------------------------------------------------------------------+
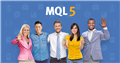
void SetIndexStyle( int index, // line index int type, // line type int style=EMPTY, // line style int width=EMPTY, // line width color clr=clrNONE // line color );

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
can any one help me change the code so I can permanently modify the colours thanks