
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello,
I'm new to programing and I need some guidance on a indicator modifying. I'm trying to write my own indicator but it's going very slow for now.
So my problem is - i'm editing "Daily Pivot Points shifted for different day start time" posted by Jellybean (i've added Camarilla equation and some stile options) but i have 2 problems that i cant find solution to:
1. When the day changes the levels for the previous days remain BUT the labels are deleted - I like them to remain on the chart. I just cant figure what function deletes them.
2. Problem that i have is - I like to show the price at the levels names - like it shows on the picture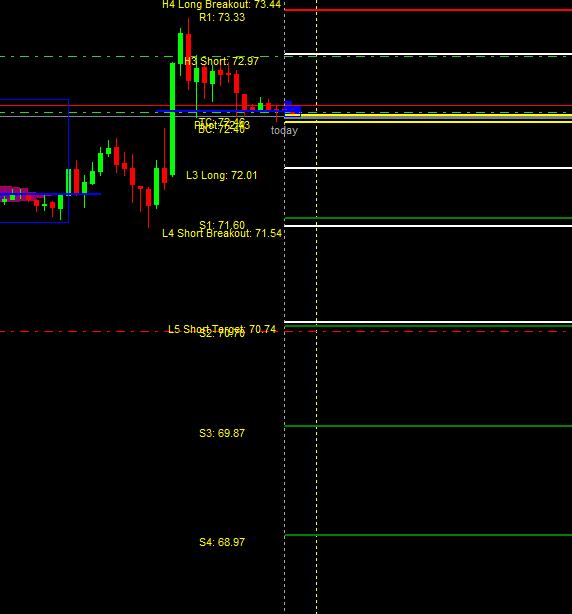
I'm adding my modified code
THANK YOU FOR YOUR TIME AND HELP.
Have a nice day/evening and happy life.