I would like help with this EA, when I set the "Risk%" parameter of this EA it opens 2.00K orders in the index. I am testing with 1% risk, but regardless of the value of risk and the value of the balance it always opens 2.00K, I will leave below the part of the code that deals with "Risk%", please if someone can help!!!
Arguably, you'd want to round-down to the next volume_step so you wouldn't exceed your risk calculations. The formula to do so is
double volume_step=SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_STEP); double lots_rounded_down_to_step = volume_step * floor(volume / volume_step);
I made that change you said but did not hear changes, below I leave a picture showing the lot size, the balance value for backtesting is 1000 and the risk is 1%, but EA keeps opening 2.00K. Noting that in the index market the minimum lot size is 1.0
Tôi đã thực hiện thay đổi mà bạn nói nhưng không thấy những thay đổi, dưới đây tôi để lại một hình ảnh cho thấy rất nhiều kích thước, cân bằng giá trị cho backtesting là 1000 và cơ sở là 1%, nhưng EA tiếp continue opening 2.00K. Lưu ý rằng trong cực đại chỉ thị nhà thầu tối thiểu là 1.0
it.
Your error is obviously in your upstream code, and nobody can help you because you haven't posted it.
The original code of this EA is here: https://www.mql5.com/en/code/19498
If you prefer to put the code here I will leave it whole below from the input parameters and exactly how I configured my parameters
//--- input parameters input bool InpLotManual = false; // Lots manual input double InpLots = 1.0; // Lots input double Risk = 1; // Risk in percent from a free margin (if "Lots manual" == false) input ushort InpStopLoss = 0; // Stop Loss (in pips) input ushort InpTakeProfit = 30; // Take Profit (in pips) input bool InpCloseBySignal = true; // Close by signal input string _DailyBreak = " --- Daily Break ---"; input ushort InpDB_BreakPoint = 20; // "Daily Break" Break point (in pips) input ushort InpDB_LastBarSizeMin = 5; // "Daily Break" Last bar size min (in pips) input ushort InpDB_LastBarSizeMax = 50; // "Daily Break" Last bar size max (in pips) input ushort InpDB_TrailingStop = 2; // "Daily Break" Trailing stop (in pips) input ushort InpDB_TrailingStep = 2; // "Daily Break" Trailing step (in pips) input ulong m_magic=26068944;// magic number //--- ulong m_slippage=30; // slippage double ExtStopLoss=0.0; double ExtTakeProfit=0.0; double ExtDB_BreakPoint=0.0; double ExtDB_LastBarSizeMin=0.0; double ExtDB_LastBarSizeMax=0.0; double ExtDB_TrailingStop=0.0; double ExtDB_TrailingStep=0.0; double m_adjusted_point; // point value adjusted for 3 or 5 points //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- if(!m_symbol.Name(Symbol())) // sets symbol name return(INIT_FAILED); RefreshRates(); if(InpLotManual) { string err_text=""; if(!CheckVolumeValue(InpLots,err_text)) { Print(err_text); return(INIT_PARAMETERS_INCORRECT); } } //--- m_trade.SetExpertMagicNumber(m_magic); //--- if(IsFillingTypeAllowed(SYMBOL_FILLING_FOK)) m_trade.SetTypeFilling(ORDER_FILLING_FOK); else if(IsFillingTypeAllowed(SYMBOL_FILLING_IOC)) m_trade.SetTypeFilling(ORDER_FILLING_IOC); else m_trade.SetTypeFilling(ORDER_FILLING_RETURN); //--- m_trade.SetDeviationInPoints(m_slippage); //--- tuning for 3 or 5 digits int digits_adjust=1; if(m_symbol.Digits()==3 || m_symbol.Digits()==5) digits_adjust=10; m_adjusted_point=m_symbol.Point()*digits_adjust; ExtStopLoss = InpStopLoss * m_adjusted_point; ExtTakeProfit = InpTakeProfit * m_adjusted_point; ExtDB_BreakPoint = InpDB_BreakPoint * m_adjusted_point; ExtDB_LastBarSizeMin = InpDB_LastBarSizeMin * m_adjusted_point; ExtDB_LastBarSizeMax = InpDB_LastBarSizeMax * m_adjusted_point; ExtDB_TrailingStop = InpDB_TrailingStop * m_adjusted_point; ExtDB_TrailingStep = InpDB_TrailingStep * m_adjusted_point; //--- if(!InpLotManual) { if(!m_money.Init(GetPointer(m_symbol),Period(),m_symbol.Point()*digits_adjust)) return(INIT_FAILED); m_money.Percent(Risk); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- static datetime NewTime=0; bool _isNewBar=false; bool _isBullBar=false; if(NewTime!=iTime(0)) { NewTime=iTime(0); _isNewBar=true; } double DayOpen = iOpen(1,m_symbol.Name(),PERIOD_D1); double last_open = iOpen(1); double last_close = iClose(1); double last_high = iHigh(1); if(last_close>last_open) _isBullBar=true; else _isBullBar=false; //--- Strategy Daily Break Point if(_isNewBar) { if(!RefreshRates()) { NewTime=iTime(1); return; } double BreakBuy = DayOpen+ExtDB_BreakPoint; double BreakSell= DayOpen-ExtDB_BreakPoint; if(_isBullBar && m_symbol.Bid()-DayOpen>=ExtDB_BreakPoint && last_close-last_open<=ExtDB_LastBarSizeMax && last_close-last_open>=ExtDB_LastBarSizeMin && BreakBuy>=last_open && BreakBuy<=last_close) { if(InpCloseBySignal) { ClosePositions(POSITION_TYPE_BUY); double sl=(InpStopLoss==0)?0.0:m_symbol.Bid()+ExtStopLoss; double tp=(InpTakeProfit==0)?0.0:m_symbol.Bid()-ExtTakeProfit; OpenSell(sl,tp); } else { double sl=(InpStopLoss==0)?0.0:m_symbol.Ask()-ExtStopLoss; double tp=(InpTakeProfit==0)?0.0:m_symbol.Ask()+ExtTakeProfit; OpenBuy(sl,tp); } } if(!_isBullBar && DayOpen-m_symbol.Ask()>=ExtDB_BreakPoint && last_open-last_close<=ExtDB_LastBarSizeMax && last_open-last_close>=ExtDB_LastBarSizeMin && BreakSell<=last_open && BreakSell>=last_close) { if(InpCloseBySignal) { ClosePositions(POSITION_TYPE_SELL); double sl=(InpStopLoss==0)?0.0:m_symbol.Ask()-ExtStopLoss; double tp=(InpTakeProfit==0)?0.0:m_symbol.Ask()+ExtTakeProfit; OpenBuy(sl,tp); } else { double sl=(InpStopLoss==0)?0.0:m_symbol.Bid()+ExtStopLoss; double tp=(InpTakeProfit==0)?0.0:m_symbol.Bid()-ExtTakeProfit; OpenSell(sl,tp); } } } //--- if(!RefreshRates()) return; Trailing(); } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //--- } //+------------------------------------------------------------------+ //| Trailing | //+------------------------------------------------------------------+ void Trailing() { double ExtTrailingStop=ExtDB_TrailingStop; double ExtTrailingStep=ExtDB_TrailingStep; for(int i=PositionsTotal()-1;i>=0;i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.Symbol()==m_symbol.Name() && m_position.Magic()==m_magic) { if(m_position.PositionType()==POSITION_TYPE_BUY) { if(m_position.PriceCurrent()-m_position.PriceOpen()>ExtTrailingStop+ExtTrailingStep) if(m_position.StopLoss()<m_position.PriceCurrent()-(ExtTrailingStop+ExtTrailingStep)) { if(!m_trade.PositionModify(m_position.Ticket(), m_symbol.NormalizePrice(m_position.PriceCurrent()-ExtTrailingStop), m_position.TakeProfit())) Print("Modify ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); continue; } } else { if(m_position.PriceOpen()-m_position.PriceCurrent()>ExtTrailingStop+ExtTrailingStep) if((m_position.StopLoss()>(m_position.PriceCurrent()+(ExtTrailingStop+ExtTrailingStep))) || (m_position.StopLoss()==0)) { if(!m_trade.PositionModify(m_position.Ticket(), m_symbol.NormalizePrice(m_position.PriceCurrent()+ExtTrailingStop), m_position.TakeProfit())) Print("Modify ",m_position.Ticket(), " Position -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); } } } } //+------------------------------------------------------------------+ //| Refreshes the symbol quotes data | //+------------------------------------------------------------------+ bool RefreshRates(void) { //--- refresh rates if(!m_symbol.RefreshRates()) { Print("RefreshRates error"); return(false); } //--- protection against the return value of "zero" if(m_symbol.Ask()==0 || m_symbol.Bid()==0) return(false); //--- return(true); } //+------------------------------------------------------------------+ //| Check the correctness of the order volume | //+------------------------------------------------------------------+ bool CheckVolumeValue(double volume,string &error_description) { //--- minimal allowed volume for trade operations // double min_volume=m_symbol.LotsMin(); double min_volume=SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MIN); if(volume<min_volume) { error_description=StringFormat("Volume is less than the minimal allowed SYMBOL_VOLUME_MIN=%.2f",min_volume); return(false); } //--- maximal allowed volume of trade operations // double max_volume=m_symbol.LotsMax(); double max_volume=SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MAX); if(volume>max_volume) { error_description=StringFormat("Volume is greater than the maximal allowed SYMBOL_VOLUME_MAX=%.2f",max_volume); return(false); } //--- get minimal step of volume changing // double volume_step=m_symbol.LotsStep(); double volume_step=SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_STEP); int ratio=(int)MathRound(volume/volume_step); if(MathAbs(ratio*volume_step-volume)>0.0000001) { error_description=StringFormat("Volume is not a multiple of the minimal step SYMBOL_VOLUME_STEP=%.2f, the closest correct volume is %.2f", volume_step,ratio*volume_step); return(false); } error_description="Correct volume value"; return(true); } //+------------------------------------------------------------------+ //| Checks if the specified filling mode is allowed | //+------------------------------------------------------------------+ bool IsFillingTypeAllowed(int fill_type) { //--- Obtain the value of the property that describes allowed filling modes int filling=m_symbol.TradeFillFlags(); //--- Return true, if mode fill_type is allowed return((filling & fill_type)==fill_type); } //+------------------------------------------------------------------+ //| Get Open for specified bar index | //+------------------------------------------------------------------+ double iOpen(const int index,string symbol=NULL,ENUM_TIMEFRAMES timeframe=PERIOD_CURRENT) { if(symbol==NULL) symbol=m_symbol.Name(); if(timeframe==0) timeframe=Period(); double Open[1]; double open=0; int copied=CopyOpen(symbol,timeframe,index,1,Open); if(copied>0) open=Open[0]; return(open); } //+------------------------------------------------------------------+ //| Get the High for specified bar index | //+------------------------------------------------------------------+ double iHigh(const int index,string symbol=NULL,ENUM_TIMEFRAMES timeframe=PERIOD_CURRENT) { if(symbol==NULL) symbol=m_symbol.Name(); if(timeframe==0) timeframe=Period(); double High[1]; double high=0; int copied=CopyHigh(symbol,timeframe,index,1,High); if(copied>0) high=High[0]; return(high); } //+------------------------------------------------------------------+ //| Get Close for specified bar index | //+------------------------------------------------------------------+ double iClose(const int index,string symbol=NULL,ENUM_TIMEFRAMES timeframe=PERIOD_CURRENT) { if(symbol==NULL) symbol=m_symbol.Name(); if(timeframe==0) timeframe=Period(); double Close[1]; double close=0; int copied=CopyClose(symbol,timeframe,index,1,Close); if(copied>0) close=Close[0]; return(close); } //+------------------------------------------------------------------+ //| Get Time for specified bar index | //+------------------------------------------------------------------+ datetime iTime(const int index,string symbol=NULL,ENUM_TIMEFRAMES timeframe=PERIOD_CURRENT) { if(symbol==NULL) symbol=m_symbol.Name(); if(timeframe==0) timeframe=Period(); datetime Time[1]; datetime time=0; int copied=CopyTime(symbol,timeframe,index,1,Time); if(copied>0) time=Time[0]; return(time); } //+------------------------------------------------------------------+ //| Open Buy position | //+------------------------------------------------------------------+ void OpenBuy(double sl,double tp) { sl=m_symbol.NormalizePrice(sl); tp=m_symbol.NormalizePrice(tp); double check_open_long_lot=0.0; if(!InpLotManual) { check_open_long_lot=m_money.CheckOpenLong(m_symbol.Ask(),sl); if(check_open_long_lot==0.0) return; } else check_open_long_lot=InpLots; //--- check volume before OrderSend to avoid "not enough money" error (CTrade) double check_volume_lot=m_trade.CheckVolume(m_symbol.Name(),check_open_long_lot,m_symbol.Ask(),ORDER_TYPE_BUY); if(check_volume_lot!=0.0) if(check_volume_lot>=check_open_long_lot) { if(m_trade.Buy(check_open_long_lot,NULL,m_symbol.Ask(),sl,tp)) { if(m_trade.ResultDeal()==0) { Print("#1 Buy -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } else { Print("#2 Buy -> true. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } } else { Print("#3 Buy -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } } //--- } //+------------------------------------------------------------------+ //| Open Sell position | //+------------------------------------------------------------------+ void OpenSell(double sl,double tp) { sl=m_symbol.NormalizePrice(sl); tp=m_symbol.NormalizePrice(tp); double check_open_short_lot=0.0; if(!InpLotManual) { check_open_short_lot=m_money.CheckOpenShort(m_symbol.Bid(),sl); if(check_open_short_lot==0.0) return; } //--- check volume before OrderSend to avoid "not enough money" error (CTrade) double check_volume_lot=m_trade.CheckVolume(m_symbol.Name(),check_open_short_lot,m_symbol.Bid(),ORDER_TYPE_SELL); if(check_volume_lot!=0.0) if(check_volume_lot>=check_open_short_lot) { if(m_trade.Sell(check_open_short_lot,NULL,m_symbol.Bid(),sl,tp)) { if(m_trade.ResultDeal()==0) { Print("#1 Sell -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } else { Print("#2 Sell -> true. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } } else { Print("#3 Sell -> false. Result Retcode: ",m_trade.ResultRetcode(), ", description of result: ",m_trade.ResultRetcodeDescription()); //PrintResult(m_trade,m_symbol); } } //--- } //+------------------------------------------------------------------+ //| Close positions | //+------------------------------------------------------------------+ void ClosePositions(const ENUM_POSITION_TYPE pos_type) { for(int i=PositionsTotal()-1;i>=0;i--) // returns the number of current positions if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties if(m_position.Symbol()==Symbol() && m_position.Magic()==m_magic) if(m_position.PositionType()==pos_type) // gets the position type m_trade.PositionClose(m_position.Ticket()); // close a position by the specified symbol } //+------------------------------------------------------------------+
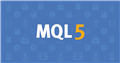
- votes: 19
- 2018.01.22
- Vladimir Karputov
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would like help with this EA, when I set the "Risk%" parameter of this EA it opens 2.00K orders in the index. I am testing with 1% risk, but regardless of the value of risk and the value of the balance it always opens 2.00K, I will leave below the part of the code that deals with "Risk%", please if someone can help!!!