Great Indicator ! I created the same using buffers, any idea on how to plot support and resistance levels for each day, instead of having only the current day ?
Here's my version so far. But I can't find a way to get 10 days of daily bars for each 'current timeframe' bar..
//+------------------------------------------------------------------+ //| B_ATR_Levels.mq5 | //+------------------------------------------------------------------+ #property copyright "Skullnick" #property link "https://www.mql5.com" #property version "1.00" //--- Indicator Plot Settings #property indicator_chart_window #property indicator_buffers 6 #property indicator_plots 6 #property indicator_type1 DRAW_LINE #property indicator_color1 clrDeepSkyBlue #property indicator_width1 1 #property indicator_label1 "Resistance 1" #property indicator_style1 STYLE_DASH #property indicator_type2 DRAW_LINE #property indicator_color2 clrDeepSkyBlue #property indicator_width2 1 #property indicator_label2 "Resistance 2" #property indicator_style2 STYLE_DASH #property indicator_type3 DRAW_LINE #property indicator_color3 clrDeepSkyBlue #property indicator_width3 1 #property indicator_label3 "Resistance 3" #property indicator_style3 STYLE_DASH #property indicator_type4 DRAW_LINE #property indicator_color4 clrOrangeRed #property indicator_width4 1 #property indicator_label4 "Support 1" #property indicator_style4 STYLE_DASH #property indicator_type5 DRAW_LINE #property indicator_color5 clrOrangeRed #property indicator_width5 1 #property indicator_label5 "Support 2" #property indicator_style5 STYLE_DASH #property indicator_type6 DRAW_LINE #property indicator_color6 clrOrangeRed #property indicator_width6 1 #property indicator_label6 "Support 3" #property indicator_style6 STYLE_DASH //--- Inputs input int inpAtrPeriod = 21; // ATR period //--- Indicator buffers double ExtR1Buffer[]; double ExtR2Buffer[]; double ExtR3Buffer[]; double ExtS1Buffer[]; double ExtS2Buffer[]; double ExtS3Buffer[]; // Weights for Average True Range double dWeights[]; // Global Variable double dATR = 0; double dPreviousClose = 0; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,ExtR1Buffer, INDICATOR_DATA); // Resistance Level 1 SetIndexBuffer(1,ExtR2Buffer, INDICATOR_DATA); // Resistance Level 2 SetIndexBuffer(2,ExtR3Buffer, INDICATOR_DATA); // Resistance Level 3 SetIndexBuffer(3,ExtS1Buffer, INDICATOR_DATA); // Support Level 1 SetIndexBuffer(4,ExtS2Buffer, INDICATOR_DATA); // Support Level 2 SetIndexBuffer(5,ExtS3Buffer, INDICATOR_DATA); // Support Level 3 ArraySetAsSeries(ExtR1Buffer, true); ArraySetAsSeries(ExtR2Buffer, true); ArraySetAsSeries(ExtR3Buffer, true); ArraySetAsSeries(ExtS1Buffer, true); ArraySetAsSeries(ExtS2Buffer, true); ArraySetAsSeries(ExtS3Buffer, true); // Resize Weights ArrayResize(dWeights, inpAtrPeriod); // Set Smoothing double dSmoothing = 2.0 / (ArraySize(dWeights) + 1); // Weights Calculation for(int i = 0; i < ArraySize(dWeights); i++) dWeights[i] = dSmoothing * MathPow(1 - dSmoothing, i); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- // Get Daily Data and Check for Sufficient Data MqlRates oDailyRates[]; ArraySetAsSeries(oDailyRates, true); int iLast = 0; if(prev_calculated == 0) iLast = rates_total - 1; else iLast = rates_total - prev_calculated; //--- Calculate Levels for(int i = iLast; i >= 0 && !IsStopped();i--) { int iNumDaysCopied = CopyRates(_Symbol, PERIOD_D1, 1, inpAtrPeriod + 1, oDailyRates); if(iNumDaysCopied >= (inpAtrPeriod+1) && bIsNewDay() == true) { // Calculate ATR for the day dATR = dCalculateATR(oDailyRates); dPreviousClose = oDailyRates[0].close; } ExtR1Buffer[i] = dPreviousClose + 0.5 * dATR; ExtR2Buffer[i] = dPreviousClose + 0.75 * dATR; ExtR3Buffer[i] = dPreviousClose + 1.0 * dATR; ExtS1Buffer[i] = dPreviousClose - 0.5 * dATR; ExtS2Buffer[i] = dPreviousClose - 0.75 * dATR; ExtS3Buffer[i] = dPreviousClose - 1.0 * dATR; } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Calculate ATR | //+------------------------------------------------------------------+ double dCalculateATR(const MqlRates &oRates[]) { double dValue = 0; int iIndex = 0; for (int i = 0; i < ArraySize(dWeights); i++) { double dHL = oRates[i].high - oRates[i].low; double dHCp = MathAbs(oRates[i].high - oRates[i+1].close); double dLCp = MathAbs(oRates[i].low - oRates[i+1].close); double dTR = MathMax(MathMax(dHL, dHCp),dLCp); dValue += dTR * dWeights[iIndex]; iIndex++; } return dValue; } //----------------------------- // -- Check New Day //----------------------------- bool bIsNewDay(void) { bool bNewDay = false; static datetime oDateOld; datetime oDateNew[1]; MqlDateTime oStructDateOld; MqlDateTime oStructDateNew; // Copy Array of Current Time to New_Time int iCopyHandle = CopyTime(_Symbol, PERIOD_D1, 0, 1, oDateNew); TimeToStruct(oDateNew[0] , oStructDateNew); TimeToStruct(oDateOld , oStructDateOld); // If Successful copy if(iCopyHandle>0) { // If New Day if(oStructDateNew.day != oStructDateOld.day) { bNewDay = true; oDateOld = oDateNew[0]; } } return bNewDay; }
i would like a mt4 version of this please.
How to change the indicator horizontal line into a short horizontal line, showing only the right side.
xy618z #:
How to change the indicator horizontal line into a short horizontal line, showing only the right side.
How to change the indicator horizontal line into a short horizontal line, showing only the right side.
Hi
you can try to create horizontal line using
ObjectCreate(0,_name,OBJ_HLINE,0,0,0);
and start time + 'n' periods as stop time.
Anil Varma #:
Hi
you can try to create horizontal line using
and start time + 'n' periods as stop time.
Horizontal line always expands on the whole chart - you can not limit it by time parameter : https://www.mql5.com/en/docs/constants/objectconstants/enum_object/obj_hline
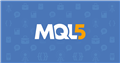
Documentation on MQL5: Constants, Enumerations and Structures / Objects Constants / Object Types / OBJ_HLINE
- www.mql5.com
OBJ_HLINE - Object Types - Objects Constants - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
ATR Probability Levels:
Probability levels based on ATR. "Probability" is calculated based on the projected Average True Range and previous period Close.
Defaults are using daily period, but any time frame supported by MetaTrader 5 can be used.
Author: Mladen Rakic