Dont use same variable names for parameters and members.
this.Market = Market;
wont work, because what the compiler actually sees here is
this.Market=this.Market;
you dot need "this" anyway, its just useful for passing and comparing pointers.
Hi Guys!
I want to create a class in MQL4, but when I compile it, launch some errors:
//+-------------------------------------------------------------------+
//| Classes |
//+-------------------------------------------------------------------+
class Trade
{
public:
string Market;
int Risk_Aversion;
string SymbolCode;
string Side;
string Order_Type;
double Entry_Price;
double Take_Profit_Price;
double Stop_Loss_Price;
int Unique_Id;
int Ticket;
Trade(string Market, string Risk_Aversion, string SymbolCode, string Side, string Order_Type, double Entry_Price, double Take_Profit_Price, double Stop_Loss_Price, int Unique_Id);
//Trade (string, string, string, string, string, double, double, double, int);
};
Trade::Trade(string Market, string Risk_Aversion, string SymbolCode, string Side, string Order_Type, double Entry_Price, double Take_Profit_Price, double Stop_Loss_Price, int Unique_Id)
{
this.Market = Market;
this.Risk_Aversion = int.Parse(Risk_Aversion);
this.SymbolCode = SymbolCode;
this.Side = Side;
this.Order_Type = Order_Type;
this.Entry_Price = Entry_Price;
this.Take_Profit_Price = Take_Profit_Price;
this.Stop_Loss_Price = Stop_Loss_Price;
this.Unique_Id = Unique_Id;
}
You may also use initialization list for constructor:
https://www.mql5.com/en/docs/basis/types/classes#initialization_list
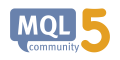
- www.mql5.com
Dont use same variable names for parameters and members.
this.Market = Market;
wont work, because what the compiler actually sees here is
this.Market=this.Market;
you dot need "this" anyway, its just useful for passing and comparing pointers.
Are you sure that a member masks the parameter? That would be a bug then. It is quite commonly used in the other OOP languages.
The only error you have to fix (technically) is this:
this.Risk_Aversion = StringToInteger(Risk_Aversion);
The others are warnings. But overall more design questions remain.
It's not a bug, the language can define whatever it wants. You ask "are you sure." That is confusion, thus is bad style.
In that function you must use this.member to differentiate from the parameter, where as in other functions the "this." is necessary.
A good style eliminates the problem. I use the following:
- Parameters and members are local variables, use lower camel case. For member variables a prefix (m or my) disambiguate the two. (For static member variables I use s or our.)
- I use upper camel case (Pascal Case) for types (no need for the c/C prefix.)
- Function names I use lower case underline separated.
It's not a bug, the language can define whatever it wants. You ask "are you sure." That is confusion, thus is bad style.
In that function you must use this.member to differentiate from the parameter, where as in other functions the "this." is necessary.
A good style eliminates the problem. I use the following:
- Parameters and members are local variables, use lower camel case. For member variables a prefix (m or my) disambiguate the two. (For static member variables I use s or our.)
- I use upper camel case (Pascal Case) for types (no need for the c/C prefix.)
- Function names I use lower case underline separated.
The above syntax for assigning the argument to a member is widely used in Java, and nobody would call it a bad style, in fact it is a recommended style. It works for MQL as well, although it generates the confusing warning. The style is not good or bad, it is an agreement of a group of people to keep their code uniform and mutually readable. So you personally can have any style you prefer, and I do not care unless we work together on the same source.
The above syntax for assigning the argument to a member is widely used in Java, and nobody would call it a bad style, in fact it is a recommended style. It works for MQL as well, although it generates the confusing warning.
The problem with this style that it produces the warnings which could bury some other important warnings (and this is bad), and MQ does not provide any means to switch off specific warning in specific code fragment.
It's not a bug, the language can define whatever it wants. You ask "are you sure." That is confusion, thus is bad style.
In that function you must use this.member to differentiate from the parameter, where as in other functions the "this." is necessary.
A good style eliminates the problem. I use the following:
- Parameters and members are local variables, use lower camel case. For member variables a prefix (m or my) disambiguate the two. (For static member variables I use s or our.)
- I use upper camel case (Pascal Case) for types (no need for the c/C prefix.)
- Function names I use lower case underline separated.
If you go by the standards set by MQ (in all of their std lib codebase)
//variables snake_case int num_one; //members begin with m_ int m_num_one;
// methods/functions/objects/classes CamelCase CExpert ExtExpert; ExtExpert.OnTick(); // unless object is a member CObject m_object;
The problem with this style that it produces the warnings which could bury some other important warnings (and this is bad), and MQ does not provide any means to switch off specific warning in specific code fragment.
True, and I have several more warnings I would love to supress (e.g. zero-length structure, no #import declaration).

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi Guys!
I want to create a class in MQL4, but when I compile it, launch some errors:
//+-------------------------------------------------------------------+
//| Classes |
//+-------------------------------------------------------------------+
class Trade
{
public:
string Market;
int Risk_Aversion;
string SymbolCode;
string Side;
string Order_Type;
double Entry_Price;
double Take_Profit_Price;
double Stop_Loss_Price;
int Unique_Id;
int Ticket;
Trade(string Market, string Risk_Aversion, string SymbolCode, string Side, string Order_Type, double Entry_Price, double Take_Profit_Price, double Stop_Loss_Price, int Unique_Id);
//Trade (string, string, string, string, string, double, double, double, int);
};
Trade::Trade(string Market, string Risk_Aversion, string SymbolCode, string Side, string Order_Type, double Entry_Price, double Take_Profit_Price, double Stop_Loss_Price, int Unique_Id)
{
this.Market = Market;
this.Risk_Aversion = int.Parse(Risk_Aversion);
this.SymbolCode = SymbolCode;
this.Side = Side;
this.Order_Type = Order_Type;
this.Entry_Price = Entry_Price;
this.Take_Profit_Price = Take_Profit_Price;
this.Stop_Loss_Price = Stop_Loss_Price;
this.Unique_Id = Unique_Id;
}