i am dealing with very low values, like: 0.0000001 vs -0.0000001.
in comparison with zero the compiler seems to be confused.
is there any way to detect precisely (and not by comparison with zero) - which number is positive and negative.
it may sound stupid... still, it is not... please help !
regards
I believe this must work ...
if your number is a, define it a as double, Not Integer (int).
then,
string result = "Neutral"; if(a>0) result = "Positive"; if(a<0) result = "Negative";
You can also try to use a string value to detect the negative numbers and use the "-" negative sign symbol for detection.
For example, this code uses the StringSubstr(); function to select the first symbol in the string and uses it for a reference.
double dMyAlmostZeroValue = 0; { // Your code. dMyAlmostZeroValue = -0.0000001; } string sTextValue = dMyAlmostZeroValue; sTextValue = StringSubstr(sTextValue , 0, 1); // First symbol in the string. if(sTextValue == "-" ) { Alert("Value is negative"); }
Your quote is: "in comparison with zero the compiler seems to be confused"
A "double" type variable has 15 significant digits. The compiler should be able to detect if a very small number (close to zero) is positive or negative.
-0.0000001 has only 7 digits.
https://www.mql5.com/en/docs/basis/types/double
Which variable type do you use?
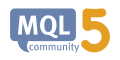
- www.mql5.com
i have them defined as doubles.
i like the idea with string (-) detection... but, really ?!!?!
Your quote is: "in comparison with zero the compiler seems to be confused"
A "double" type variable has 15 significant digits. The compiler should be able to detect if a very small number (close to zero) is positive or negative.
-0.0000001 has only 7 digits.
https://www.mql5.com/en/docs/basis/types/double
Which variable type do you use?
you seem to have had a tough confrontation with this issue before (correct?)... two posts with two good ideas.
i have them as doubles and they are not recognized vs 0 comparison.
thank you sir.
A different simple solution is multiply your small number with a large number.
Then the number of digits is smaller.
For example
-0.0000001 X 1.000.000 = -0.1
Now you can compare this number with zero as mentioned in the second post.
A different simple solution is multiply your small number with a large number.
Then the number of digits is smaller.
For example
-0.0000001 X 1.000.000 = -0.1
Now you can compare this number with zero as mentioned in the second post.
that i done. not sure if works well...
it is smthing like: if (MAArray[1]*1000000 >0){bla}
you seem to have had a tough confrontation with this issue before (correct?)... two posts with two good ideas.
i have them as doubles and they are not recognized vs 0 comparison.
thank you sir.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
i am dealing with very low values, like: 0.0000001 vs -0.0000001.
in comparison with zero the compiler seems to be confused.
is there any way to detect precisely (and not by comparison with zero) - which number is positive and negative.
it may sound stupid... still, it is not... please help !
regards