- Close By - Trade - MetaTrader 5 for Android
- Close By - Trade - MetaTrader 5 for iPhone
- Relative Vigor Index - Oscillators - Technical Indicators - Price Charts, Technical and Fundamental Analysis
This function should return the highest price between the last two closed trades, i.e. from the open of the second last trade to the close of the very last trade. I don't know where I am going wrong. Help me out.
Hi,
You can save the results of each trading position into an array with type double.
After that, you sort the numbers in the array, and take the highest or lowest number according to your needs.
Regards.
Hi,
You can save the results of each trading position into an array with type double.
After that, you sort the numbers in the array, and take the highest or lowest number according to your needs.
Regards.
Hello. Thanks for the feedback.
The highest price returned should include all the prices during when the two trades were open. So if the two trades lasted for 3 hours, (from the open of the second last trade to the close of the last trade), the highest price during these three hours is what I want.
Thanks.
When asking where you went wrong I would always answer: stop working with highly nested functions like you did, instead divide the functionality in multiple subfunctions, then you can check and correct them modularly. Because right now you are searching for a needle in a haystack.
Okay. Let's assume then that I can get the ENTRY price of the second last closed trade and the EXIT price of the very last trade comfortably, in different sub-functions. How do you propose I should write up the function that returns the highest price formed from the entry price of the second last closed trade to the exit price of the last trade? I think that's where my main problem is.
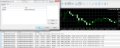
- www.mql5.com
Okay. Let's assume then that I can get the ENTRY price of the second last closed trade and the EXIT price of the very last trade comfortably, in different sub-functions. How do you propose I should write up the function that returns the highest price formed from the entry price of the second last closed trade to the exit price of the last trade? I think that's where my main problem is.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use