- Change Indicator Line Color(Repeated Question After 5 Years)
- Indicators with alerts/signal
- What to do when Data window shows different value then the iCustom value?
Hey guys, so i did research on how to accomplish this and saw some posts in the forum but for the case where color changes with each candle no line appear I guess it's because I set 2 buffers with different colors. even indices are filled in one buffer and od buffers are filled in one. In data waindow, it shows well the value but i guess because the 2 buffers have different colors the lines don't connect
+ //| RSI.mq4 | //| Copyright 2005-2014, MetaQuotes Software Corp. | //| http://www.mql4.com | //+------------------------------------------------------------------+ #property copyright "2005-2014, MetaQuotes Software Corp." #property link "http://www.mql4.com" #property description "Relative Strength Index" #property strict #property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 100 #property indicator_buffers 2 #property indicator_color1 DodgerBlue #property indicator_color2 Red //#property indicator_color3 Red #property indicator_level1 30.0 #property indicator_level2 70.0 #property indicator_levelcolor clrSilver #property indicator_levelstyle STYLE_DOT //--- input parameters input int InpRSIPeriod=14; // RSI Period //--- buffers double ExtRSIBuffer[]; double ExtPosBuffer[]; double ExtNegBuffer[]; double valda[]; double valdb[]; double valc[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit(void) { if(TimeCurrent() >= D'2024.02.21 20:00') {Alert("Not AUTHORIZED");return -1;} MathSrand(GetTickCount()); string short_name; //--- 2 additional buffers are used for counting. IndicatorBuffers(5); SetIndexBuffer(2,ExtPosBuffer); SetIndexBuffer(3,ExtNegBuffer); SetIndexBuffer(1,valda); //SetIndexBuffer(2,valdb); SetIndexBuffer(4,valc); //--- indicator line SetIndexStyle(0,DRAW_LINE); SetIndexStyle(1,DRAW_LINE); SetIndexBuffer(0,ExtRSIBuffer); //--- name for DataWindow and indicator subwindow label short_name="RSI("+string(InpRSIPeriod)+")"; IndicatorShortName(short_name); SetIndexLabel(0,short_name); //--- check for input if(InpRSIPeriod<2) { Print("Incorrect value for input variable InpRSIPeriod = ",InpRSIPeriod); return(INIT_FAILED); } //--- SetIndexDrawBegin(0,InpRSIPeriod); //--- initialization done return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Relative Strength Index | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int i,pos; double diff; if(TimeCurrent() >= D'2024.02.21 20:00') {Alert("Not AUTHORIZED");return -1;} //--- if(Bars<=InpRSIPeriod || InpRSIPeriod<2) return(0); //--- counting from 0 to rates_total ArraySetAsSeries(ExtRSIBuffer,false); ArraySetAsSeries(ExtPosBuffer,false); ArraySetAsSeries(ExtNegBuffer,false); ArraySetAsSeries(valc,false); ArraySetAsSeries(valda,false); ArraySetAsSeries(valdb,false); ArraySetAsSeries(close,false); //--- preliminary calculations pos=prev_calculated-1; if(pos<=InpRSIPeriod) { //--- first RSIPeriod values of the indicator are not calculated ExtRSIBuffer[0]=0.0; ExtPosBuffer[0]=0.0; ExtNegBuffer[0]=0.0; double sump=0.0; double sumn=0.0; for(i=1; i<=InpRSIPeriod; i++) { ExtRSIBuffer[i]=0.0; ExtPosBuffer[i]=0.0; ExtNegBuffer[i]=0.0; diff=close[i]-close[i-1]; if(diff>0) sump+=diff; else sumn-=diff; } //--- calculate first visible value ExtPosBuffer[InpRSIPeriod]=sump/InpRSIPeriod; ExtNegBuffer[InpRSIPeriod]=sumn/InpRSIPeriod; if(ExtNegBuffer[InpRSIPeriod]!=0.0){ ExtRSIBuffer[InpRSIPeriod]=100.0-(100.0/(1.0+ExtPosBuffer[InpRSIPeriod]/ExtNegBuffer[InpRSIPeriod])); } else { if(ExtPosBuffer[InpRSIPeriod]!=0.0) {ExtRSIBuffer[InpRSIPeriod]=100.0;} else {ExtRSIBuffer[InpRSIPeriod]=50.0;} } //--- prepare the position value for main calculation pos=InpRSIPeriod+1; } //Alert(pos); //--- the main loop of calculations for(i=pos; i<rates_total && !IsStopped(); i++) { // double x = ((i-pos)/2.0); // //if(i == 3004)Alert(i," ",mod((i - pos)/2.0, 2.0)," ",close[i]," ",MathRound(x)," ",x); // // if(mod((i - pos)/2.0, 2.0)!=0.0 && mod((i - pos)/2.0, 2.0)!=1.0){valc[i] = 2.0;} // // if(mod((i - pos)/2.0, 2.0)==1.0){ // valc[i] = 1.0; // } // if(mod((i - pos)/2.0, 2.0)==0.0){ // valc[i] = 0.0; // } //Alert(i," ",MathMod(MathMod(i, pos),4)); bool parity = false; if(MathMod(pos, 2) == 1.0){parity = false;} if(MathMod(pos, 2) == 0.0){parity = true;} diff=close[i]-close[i-1]; ExtPosBuffer[i]=(ExtPosBuffer[i-1]*(InpRSIPeriod-1)+(diff>0.0?diff:0.0))/InpRSIPeriod; ExtNegBuffer[i]=(ExtNegBuffer[i-1]*(InpRSIPeriod-1)+(diff<0.0?-diff:0.0))/InpRSIPeriod; if(ExtNegBuffer[i]!=0.0){ //valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]); if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);} if(parity && MathMod(i, 2) == 1.0){valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);} //if(valc[i] == 1.0){} } else { if(ExtPosBuffer[i]!=0.0){ if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=100.0;} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=100.0;} if(parity && MathMod(i, 2) == 1.0){valda[i] = 100.0;} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 100.0;} }else {if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=50.0;} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=50.0;} if(parity && MathMod(i, 2) == 1.0){valda[i] = 50.0;} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 50.0;} } } } //Alert(ExtRSIBuffer[rates_total-1]," ",ExtRSIBuffer[5]); //--- return(rates_total); } //+------------------------------------------------------------------+ double mod(double value1, double value2){ if(MathRound(value1)-value1 != 0.0){ return -1; } if(MathRound(value1)-value1 == 0.0){ return MathMod(value1, value2); } return -2; }
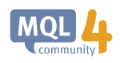
- www.mql4.com
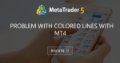
- 2023.01.26
- www.mql5.com
Same as any colored line indicator. Look in the CodeBase. If you assign to one color buffer, make the other color buffer(s) EMPTY_VALUE. Then connect to the previous bar.
HOW CAN I hide CONNECTION lines of plots? (ttt) - MQL4 programming forum (2016)
For MT4, I use:
- One buffer has the value, color set to CLR_NONE so not shown on chart, (but in data window and pop up.)
- Two buffers, one color each, with SetIndexLabel(i, NULL) so they don't show in data window.
- Then you need to connect the lines on color change. downBuffer[i]=value[i]; if(downBuffer[i+1]==EMPTY_VALUE) downBuffer[i+1]=value[i].
Same as any colored line indicator. Look in the CodeBase. If you assign to one color buffer, make the other color buffer(s) EMPTY_VALUE. Then connect to the previous bar.
HOW CAN I hide CONNECTION lines of plots? (ttt) - MQL4 programming forum (2016)
For MT4, I use:
- One buffer has the value, color set to CLR_NONE so not shown on chart, (but in data window and pop up.)
- Two buffers, one color each, with SetIndexLabel(i, NULL) so they don't show in data window.
- Then you need to connect the lines on color change. downBuffer[i]=value[i]; if(downBuffer[i+1]==EMPTY_VALUE) downBuffer[i+1]=value[i].
please, can you explain what you mean by connect? I did just that. I set one buffer value and the other I set EMPTY VALUE. In data window it shows but the values and they are correct but nothings is drawn, I was thinking maybe because it changes color every candle. I managed to do every 2 candle color chnge but every 1 candle have this issue where nothing draw. I was thinking maybe it is because 2 consecutive values are each in 2 separated buffer with different colors.
please, can you explain what you mean by connect? I did just that. I set one buffer value and the other I set EMPTY VALUE. In data window it shows but the values and they are correct but nothings is drawn, I was thinking maybe because it changes color every candle. I managed to do every 2 candle color chnge but every 1 candle have this issue where nothing draw. I was thinking maybe it is because 2 consecutive values are each in 2 separated buffer with different colors.
//+------------------------------------------------------------------+ //| RSI.mq4 | //| Copyright 2005-2014, MetaQuotes Software Corp. | //| http://www.mql4.com | //+------------------------------------------------------------------+ #property copyright "2005-2014, MetaQuotes Software Corp." #property link "http://www.mql4.com" #property description "Relative Strength Index" #property strict #property indicator_separate_window #property indicator_minimum 0 #property indicator_maximum 100 #property indicator_buffers 2 #property indicator_color1 DodgerBlue #property indicator_color2 Red //#property indicator_color3 Red #property indicator_level1 30.0 #property indicator_level2 70.0 #property indicator_levelcolor clrSilver #property indicator_levelstyle STYLE_DOT //--- input parameters input int InpRSIPeriod=14; // RSI Period //--- buffers double ExtRSIBuffer[]; double ExtPosBuffer[]; double ExtNegBuffer[]; double valda[]; double valdb[]; double valc[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit(void) { if(TimeCurrent() >= D'2024.02.21 20:00') {Alert("Not AUTHORIZED");return -1;} MathSrand(GetTickCount()); string short_name; //--- 2 additional buffers are used for counting. IndicatorBuffers(5); SetIndexBuffer(2,ExtPosBuffer); SetIndexBuffer(3,ExtNegBuffer); SetIndexBuffer(1,valda); //SetIndexBuffer(2,valdb); SetIndexBuffer(4,valc); //--- indicator line SetIndexStyle(0,DRAW_LINE); SetIndexStyle(1,DRAW_LINE); SetIndexBuffer(0,ExtRSIBuffer); //--- name for DataWindow and indicator subwindow label short_name="RSI("+string(InpRSIPeriod)+")"; IndicatorShortName(short_name); SetIndexLabel(0,short_name); //--- check for input if(InpRSIPeriod<2) { Print("Incorrect value for input variable InpRSIPeriod = ",InpRSIPeriod); return(INIT_FAILED); } //--- SetIndexDrawBegin(0,InpRSIPeriod); //--- initialization done return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Relative Strength Index | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int i,pos; double diff; if(TimeCurrent() >= D'2024.02.21 20:00') {Alert("Not AUTHORIZED");return -1;} //--- if(Bars<=InpRSIPeriod || InpRSIPeriod<2) return(0); //--- counting from 0 to rates_total ArraySetAsSeries(ExtRSIBuffer,false); ArraySetAsSeries(ExtPosBuffer,false); ArraySetAsSeries(ExtNegBuffer,false); ArraySetAsSeries(valc,false); ArraySetAsSeries(valda,false); ArraySetAsSeries(valdb,false); ArraySetAsSeries(close,false); //--- preliminary calculations pos=prev_calculated-1; if(pos<=InpRSIPeriod) { //--- first RSIPeriod values of the indicator are not calculated ExtRSIBuffer[0]=0.0; ExtPosBuffer[0]=0.0; ExtNegBuffer[0]=0.0; double sump=0.0; double sumn=0.0; for(i=1; i<=InpRSIPeriod; i++) { ExtRSIBuffer[i]=0.0; ExtPosBuffer[i]=0.0; ExtNegBuffer[i]=0.0; diff=close[i]-close[i-1]; if(diff>0) sump+=diff; else sumn-=diff; } //--- calculate first visible value ExtPosBuffer[InpRSIPeriod]=sump/InpRSIPeriod; ExtNegBuffer[InpRSIPeriod]=sumn/InpRSIPeriod; if(ExtNegBuffer[InpRSIPeriod]!=0.0){ ExtRSIBuffer[InpRSIPeriod]=100.0-(100.0/(1.0+ExtPosBuffer[InpRSIPeriod]/ExtNegBuffer[InpRSIPeriod])); } else { if(ExtPosBuffer[InpRSIPeriod]!=0.0) {ExtRSIBuffer[InpRSIPeriod]=100.0;} else {ExtRSIBuffer[InpRSIPeriod]=50.0;} } //--- prepare the position value for main calculation pos=InpRSIPeriod+1; } //Alert(pos); //--- the main loop of calculations for(i=pos; i<rates_total && !IsStopped(); i++) { // double x = ((i-pos)/2.0); // //if(i == 3004)Alert(i," ",mod((i - pos)/2.0, 2.0)," ",close[i]," ",MathRound(x)," ",x); // // if(mod((i - pos)/2.0, 2.0)!=0.0 && mod((i - pos)/2.0, 2.0)!=1.0){valc[i] = 2.0;} // // if(mod((i - pos)/2.0, 2.0)==1.0){ // valc[i] = 1.0; // } // if(mod((i - pos)/2.0, 2.0)==0.0){ // valc[i] = 0.0; // } //Alert(i," ",MathMod(MathMod(i, pos),4)); bool parity = false; if(MathMod(pos, 2) == 1.0){parity = false;} if(MathMod(pos, 2) == 0.0){parity = true;} diff=close[i]-close[i-1]; ExtPosBuffer[i]=(ExtPosBuffer[i-1]*(InpRSIPeriod-1)+(diff>0.0?diff:0.0))/InpRSIPeriod; ExtNegBuffer[i]=(ExtNegBuffer[i-1]*(InpRSIPeriod-1)+(diff<0.0?-diff:0.0))/InpRSIPeriod; if(ExtNegBuffer[i]!=0.0){ //valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]); if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);valda[i] = EMPTY_VALUE;} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);valda[i] = EMPTY_VALUE;} if(parity && MathMod(i, 2) == 1.0){valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);ExtRSIBuffer[i] = EMPTY_VALUE;} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 100.0-100.0/(1+ExtPosBuffer[i]/ExtNegBuffer[i]);ExtRSIBuffer[i] = EMPTY_VALUE;} //if(valc[i] == 1.0){} } else { if(ExtPosBuffer[i]!=0.0){ if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=100.0;} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=100.0;} if(parity && MathMod(i, 2) == 1.0){valda[i] = 100.0;} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 100.0;} }else {if(parity && MathMod(i, 2) == 0.0){ExtRSIBuffer[i]=50.0;} if(!parity && MathMod(i, 2) == 1.0){ExtRSIBuffer[i]=50.0;} if(parity && MathMod(i, 2) == 1.0){valda[i] = 50.0;} if(!parity && MathMod(i, 2) == 0.0){valda[i] = 50.0;} } } } //Alert(ExtRSIBuffer[rates_total-1]," ",ExtRSIBuffer[5]); //--- return(rates_total); } //+------------------------------------------------------------------+ double mod(double value1, double value2){ if(MathRound(value1)-value1 != 0.0){ return -1; } if(MathRound(value1)-value1 == 0.0){ return MathMod(value1, value2); } return -2; }
I added the EMPTYVALUE. But still nothing shows but on chart but data window shows the value correctly
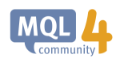
- www.mql4.com
I don't really see bro. I have the same issue as you for consecutive change of color. Nothing draws
please, can you explain what you mean by connect? I did just that. I set one buffer value and the other I set EMPTY VALUE. In data window it shows but the values and they are correct but nothings is drawn, I was thinking maybe because it changes color every candle. I managed to do every 2 candle color chnge but every 1 candle have this issue where nothing draw. I was thinking maybe it is because 2 consecutive values are each in 2 separated buffer with different colors.
the solution presented here is not for consecutive colors change
it was helpful thanks a lot

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use