ISSUE is.. One set of trade happened good.. BUY and Close, when SELL need to be done .. the SL and TP values are not even near to At price. where as at price is good as per market. sample issue trades as below
FIRST SELL TRADE and GOT CLOSED FIne.. but opposite signal BUY trade the SL and TP values are nowhere near to the symbol price..
2023.09.19 10:22:30.307 Trades '114865964': accepted instant sell 0.01 XAUUSD at 1935.355 sl: 1935.680 tp: 1934.355 (deviation: 1)
2023.09.19 10:22:30.558 Trades '114865964': deal #229981795 sell 0.01 XAUUSD at 1935.355 done (based on order #412300185)
2023.09.19 10:22:30.558 Trades '114865964': order #412300185 sell 0.01 / 0.01 XAUUSD at 1935.355 done in 483.811 ms (ok)
2023.09.19 10:24:03.502 Trades '114865964': instant buy 0.01 XAUUSD at 1935.515, close #412300185 sell 0.01 XAUUSD 1935.355 (deviation: 1)
2023.09.19 10:24:03.734 Trades '114865964': accepted instant buy 0.01 XAUUSD at 1935.515, close #412300185 sell 0.01 XAUUSD 1935.355 (deviation: 1)
2023.09.19 10:24:04.033 Trades '114865964': deal #229982701 buy 0.01 XAUUSD at 1935.515 done (based on order #412302013)
2023.09.19 10:24:04.034 Trades '114865964': order #412302013 buy 0.01 / 0.01 XAUUSD at 1935.515 done in 531.635 ms (ok)
2023.09.19 10:24:04.540 Trades '114865964': failed instant buy 0.01 XAUUSD at 1935.528 sl: -1264.597 tp: 17935.528 (deviation: 1) [Invalid stops]
request.sl = SymbolInfoDouble(Symbol(), SYMBOL_ASK) + stopLoss * _Point; // Calculate stop-loss request.tp = SymbolInfoDouble(Symbol(), SYMBOL_BID) - takeProfit * _Point; // Calculate take-profit
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Thank you @William Roeder.....
Acutally my strategy logic do not require a SL and TP. However, I do not have expertise in coding and I framed the above code with GPT.
Some brokers seems to have TP and SL to be part of the order placement... What I need actually is...
Condition - BUY ORDER
1. After a RED Heikin(HA) Candle - When a Green HA candle breaks its high, we will enter a BUY position
2. We will exit the BUY position when a new RED HA candle low breaks the low of previoud Green HA candle.. This should close the existing BUY positions. Also, this will be entry signal for the SELL Order.
3. So the SELL position will be exited after a Green HA candle breaks the HIGH of the previous RED HA candle.
Other issue I have is the rounding of decimals to the symbol value... not sure I have done it correctly.
Could you please help me out which the above changes in my code Sir?
thank you in advance !!
Regards, Gopi
Don't request help for ChatGPT (or other A.I.) generated code. It generates horrible code, mixing MQL4 and MQL5. Consider using the Freelance section for such requests.
To learn MQL programming, you can research the many available Articles on the subject, or examples in the Codebase, as well as reference the online Documentation.
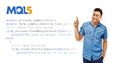
- 2023.09.19
- www.mql5.com
Hi,
What i meant is the reference I have taken across and not completely developed using it..
Hoping to get a reply from William on this.. Thank you..
@William Roeder, has already given you several points for you to address in your code. The very first one is major and critical and is the primary reason for your issue.
Attempt fixing the issue that has been pointed out, and show your new code with your alterations.
If you are not willing to do that then I will have to remove the thread, and you will have to consider using the Freelance section instead.
#property copyright "Copyright 2023, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "3.00" input double lotSize = 0.01; // Lot size for order input double stopLossPips = 100.0; // Stop loss in pips input double takeProfitPips = 1000.0; // Take profit in pips int heikenAshi; #include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> #include <Trade\AccountInfo.mqh> //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { heikenAshi = iCustom(_Symbol, _Period, "simpleHeikinAshi"); double heikenAshiOpen[], heikenAshiHigh[], heikenAshiLow[], heikenAshiClose[]; CopyBuffer(heikenAshi, 0, 0, 2, heikenAshiOpen); CopyBuffer(heikenAshi, 1, 0, 2, heikenAshiHigh); CopyBuffer(heikenAshi, 2, 0, 2, heikenAshiLow); CopyBuffer(heikenAshi, 3, 0, 2, heikenAshiClose); return(INIT_SUCCEEDED); } bool oppositeConditionTriggered = false; // Flag to track whether the opposite entry condition has been triggered int oppositeConditionCheckInterval = 5; // Check the opposite condition every 5 ticks int ticksSinceLastOppositeCheck = 0; // Counter to keep track of ticks ulong lastClosedPosition = 0; // Track the last closed position // ... (Previous code) int cooldownTicks = 1; // Cooldown period in ticks int currentCooldown = 0; // Counter for the cooldown period // ... (Previous code) bool isPositionOpen = false; // Flag to track whether a position is currently open // ... (Previous code) // ... (Previous code) void OnTick() { // Increment the tick counter ticksSinceLastOppositeCheck++; if (currentCooldown > 0) { currentCooldown--; // Decrement the cooldown counter } heikenAshi = iCustom(_Symbol, _Period, "simpleHeikinAshi"); double heikenAshiOpen[], heikenAshiHigh[], heikenAshiLow[], heikenAshiClose[]; CopyBuffer(heikenAshi, 0, 0, 2, heikenAshiOpen); CopyBuffer(heikenAshi, 1, 0, 2, heikenAshiHigh); CopyBuffer(heikenAshi, 2, 0, 2, heikenAshiLow); CopyBuffer(heikenAshi, 3, 0, 2, heikenAshiClose); // Check if a position is open if (PositionsTotal() > 0) { isPositionOpen = true; } else { isPositionOpen = false; } // Determine the type of the current position int currentPositionType = 0; // 0 for no position, 1 for Buy, -1 for Sell if (isPositionOpen) { currentPositionType = (PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY) ? 1 : -1; } // Your new trade logic here if ((heikenAshiOpen[1] < heikenAshiClose[1]) && (heikenAshiHigh[1] > heikenAshiHigh[0])) { // Buy entry condition if (!oppositeConditionTriggered && !isPositionOpen && currentCooldown == 0) { Print("Buy entry condition met."); PlaceMarketBuyOrder(); Print(Symbol(), " BUY"); oppositeConditionTriggered = true; // Set the flag to true to prevent multiple orders currentCooldown = cooldownTicks; // Start the cooldown period } } else if ((heikenAshiClose[1] > heikenAshiOpen[1]) && (heikenAshiLow[1] < heikenAshiLow[0])) { // Sell entry condition if (!oppositeConditionTriggered && !isPositionOpen && currentCooldown == 0) { Print("Sell entry condition met."); PlaceMarketSellOrder(); Print(Symbol(), " SELL"); oppositeConditionTriggered = true; // Set the flag to true to prevent multiple orders currentCooldown = cooldownTicks; // Start the cooldown period } } // Check for the opposite entry condition (exit and wait for the opposite) if (isPositionOpen && (ticksSinceLastOppositeCheck >= oppositeConditionCheckInterval)) { if (OppositeEntryCondition(currentPositionType)) { Print("Opposite entry condition triggered. Closing all positions."); CloseAllPositions(); // Close positions after waiting for some ticks oppositeConditionTriggered = true; // Set the flag to true currentCooldown = cooldownTicks; // Start the cooldown period } } // Reset the oppositeConditionTriggered flag if it was set if (oppositeConditionTriggered && !isPositionOpen) { oppositeConditionTriggered = false; } } bool OppositeEntryCondition(int currentPositionType) { // Define your logic for the opposite entry condition here // For BUY, you can check for SELL conditions, and vice versa // Return true if the opposite entry condition is met, otherwise return false heikenAshi = iCustom(_Symbol, _Period, "simpleHeikinAshi"); double heikenAshiOpen[], heikenAshiHigh[], heikenAshiLow[], heikenAshiClose[]; CopyBuffer(heikenAshi, 0, 0, 2, heikenAshiOpen); CopyBuffer(heikenAshi, 1, 0, 2, heikenAshiHigh); CopyBuffer(heikenAshi, 2, 0, 2, heikenAshiLow); CopyBuffer(heikenAshi, 3, 0, 2, heikenAshiClose); // Example: Opposite condition for BUY if (currentPositionType == 1 && (heikenAshiOpen[1] > heikenAshiClose[1]) && (heikenAshiLow[1] < heikenAshiLow[0])) { Print("Opposite condition for BUY"); return true; } // Example: Opposite condition for SELL if (currentPositionType == -1 && (heikenAshiOpen[1] < heikenAshiClose[1]) && (heikenAshiHigh[1] > heikenAshiHigh[0])) { Print("Opposite condition for SELL"); return true; } return false; } // ... (Remaining code) // ... (Remaining code) void OnTimer() { // Reset the tick counter when the timer event occurs ticksSinceLastOppositeCheck = 0; oppositeConditionTriggered = false; // Reset the flag when a new signal is generated } // Place a market buy order // Place a market buy order void PlaceMarketBuyOrder() { MqlTradeRequest request; MqlTradeResult result; // Fill in the trade request fields for a Buy order request.action = TRADE_ACTION_DEAL; // Use a valid trade action here (e.g., TRADE_ACTION_BUY) request.symbol = Symbol(); request.volume = lotSize; request.type = ORDER_TYPE_BUY; // Use ORDER_TYPE_BUY or ORDER_TYPE_SELL request.type_filling = ORDER_FILLING_FOK; request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK); // Use SYMBOL_ASK for Buy orders request.deviation = 2; // You can adjust this value // Calculate the spread in points double spread = SYMBOL_SPREAD; // Adjust the stop loss and take profit to account for the spread request.sl = SymbolInfoDouble(Symbol(), SYMBOL_ASK) - (stopLossPips + spread) * SYMBOL_POINT; // Calculate stop-loss based on bid request.tp = SymbolInfoDouble(Symbol(), SYMBOL_ASK) + (takeProfitPips - spread) * SYMBOL_POINT; // Calculate take-profit based on bid if (!OrderSend(request, result)) { PrintFormat("OrderSend error %d", GetLastError()); // If unable to send the request, output the error code } // Print information about the operation PrintFormat("retcode=%u deal=%I64u order=%I64u", result.retcode, result.deal, result.order); } // Place a market sell order void PlaceMarketSellOrder() { MqlTradeRequest request; MqlTradeResult result; // Fill in the trade request fields for a Sell order request.action = TRADE_ACTION_DEAL; // Use a valid trade action here (e.g., TRADE_ACTION_SELL) request.symbol = Symbol(); request.volume = lotSize; request.type = ORDER_TYPE_SELL; // Use ORDER_TYPE_BUY or ORDER_TYPE_SELL request.type_filling = ORDER_FILLING_FOK; request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK); // Use SYMBOL_BID for Sell orders request.deviation = 2; // You can adjust this value // Calculate the spread in points double spread = SYMBOL_SPREAD; // Adjust the stop loss and take profit to account for the spread request.sl = SymbolInfoDouble(Symbol(), SYMBOL_ASK) + (stopLossPips + spread) * SYMBOL_POINT; // Calculate stop-loss based on ask request.tp = SymbolInfoDouble(Symbol(), SYMBOL_ASK) - (takeProfitPips - spread) * SYMBOL_POINT; // Calculate take-profit based on ask if (!OrderSend(request, result)) { PrintFormat("OrderSend error %d", GetLastError()); // If unable to send the request, output the error code } // Print information about the operation PrintFormat("retcode=%u deal=%I64u order=%I64u", result.retcode, result.deal, result.order); } void CloseAllPositions() { int totalPositions = PositionsTotal(); for (int i = totalPositions - 1; i >= 0; i--) { ulong ticket = PositionGetTicket(i); MqlTradeRequest request; MqlTradeResult result; request.action = TRADE_ACTION_DEAL; int positionType = PositionGetInteger(POSITION_TYPE); if (positionType == POSITION_TYPE_BUY) { // Closing a buy position with an instant sell order at the current bid price request.type = ORDER_TYPE_SELL; request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK); // Use bid price for selling } else if (positionType == POSITION_TYPE_SELL) { // Closing a sell position with an instant buy order at the current ask price request.type = ORDER_TYPE_BUY; request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK); // Use ask price for buying } else { // Unknown position type, skip it continue; } request.symbol = Symbol(); request.volume = PositionGetDouble(POSITION_VOLUME); request.position = ticket; request.deviation = 1; // Adjust the deviation as needed request.type_filling = ORDER_FILLING_FOK; if (OrderSend(request, result) < 0) { PrintFormat("OrderSend error %d", GetLastError()); } PrintFormat("retcode=%u deal=%I64u order=%I64u", result.retcode, result.deal, result.order); } }
here is my updated code as per suggestion.. still not working...
Also my code take only BUY trades and no sell trades so far.. inspite conditions are satisfied..
My other query is.. how come the SL and TP prices are not in sync with symbol price after one set of BUY and BUY EXIT trades.. Refer below.
. 2023.09.19 10:24:04.034 Trades '114865964': order #412302013 buy 0.01 / 0.01 XAUUSD at 1935.515 done in 531.635 ms (ok)
2023.09.19 10:24:04.540 Trades '114865964': failed instant buy 0.01 XAUUSD at 1935.528 sl: -1264.597 tp: 17935.528 (deviation: 1) [Invalid stops]
here is my updated code as per suggestion.. still not working...
Also my code take only BUY trades and no sell trades so far.. inspite conditions are satisfied..
My other query is.. how come the SL and TP prices are not in sync with symbol price after one set of BUY and BUY EXIT trades.. Refer below.
. 2023.09.19 10:24:04.034 Trades '114865964': order #412302013 buy 0.01 / 0.01 XAUUSD at 1935.515 done in 531.635 ms (ok)
2023.09.19 10:24:04.540 Trades '114865964': failed instant buy 0.01 XAUUSD at 1935.528 sl: -1264.597 tp: 17935.528 (deviation: 1) [Invalid stops]
You haven't really fixed anything yet. Lets apply some basic logic questions ...
- Do you think that a stop-loss price of -1264.597 is a valid quote price?
- Do you think that a T/P of 17935.52 seems reasonable, taking into account that it is almost 10 times greater than the open price of 1935.528?
- Is a "pip" the same unit as a quote price change?
- Is the variable values for Stop Size (in pips), Spread (in points) and Quote Prices, all of the the same of units, or are they different?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use