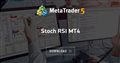
- www.mql5.com
Hei Yashar Seyyedin,
Thats true, I have seen it. the thing is that I'm trying to get it to work from within my expert advisor and not as a file include inside the ea.
One thing to mention is iHighest and iLowest return the index and can only be applied to OHLC prices not indicators.
Hei Yashar Seyyedin,
I have updated my code:
double StochRSIValue = 0.0; // Validate input parameters if (StochRSI_Period >= 2 && StochRSI_KPeriod >= 1 && StochRSI_DPeriod >= 1 && StochRSI_Slowing >= 1) { // Calculate RSI value double rsiValue = iRSI(Symbol(), 0, StochRSI_Period, PRICE_CLOSE, 0); // Calculate highest and lowest RSI values double highestRSI = -DBL_MAX; double lowestRSI = DBL_MAX; for (int i = StochRSI_Period - 1; i >= 0; i--) { double currentRSI = iRSI(Symbol(), 0, StochRSI_Period, PRICE_CLOSE, i); if (currentRSI > highestRSI) highestRSI = currentRSI; if (currentRSI < lowestRSI) lowestRSI = currentRSI; } // Calculate Stochastic Oscillator for RSI double stochRSI = 0.0; if (highestRSI != lowestRSI) { stochRSI = (rsiValue - lowestRSI) / (highestRSI - lowestRSI) * 100; } // Calculate StochRSI StochRSIValue = stochRSI; } else { Print("Invalid input parameters for StochRSI calculation."); }
I now calculate the highest and lowest RSI values within the specified StochRSI_Period and then use these values to calculate the Stochastic RSI.
Hei Yashar Seyyedin,
I have updated my code:
I now calculate the highest and lowest RSI values within the specified StochRSI_Period and then use these values to calculate the Stochastic RSI.
stochRSI value is not the end of story. You should calculate %K and %D to complete the task.
Something close to this:
kArray[i]=iMAOnArray(stochRSIArray, Bars, smoothK, 0, MODE_SMA, i); dArray[i]=iMAOnArray(kArray, Bars, smoothD, 0, MODE_SMA, i);
stochRSI value is not the end of story. You should calculate %K and %D to complete the task.
Something close to this:
Hei Yashar,
is this whatt you had in mind.
double StochRSIValue = 0.0; // Validate input parameters if (StochRSI_Period >= 2 && StochRSI_KPeriod >= 1 && StochRSI_DPeriod >= 1 && StochRSI_Slowing >= 1) { // Calculate RSI value double rsiValue = iRSI(Symbol(), 0, StochRSI_Period, PRICE_CLOSE, 0); // Calculate highest and lowest RSI values double highestRSI = -DBL_MAX; double lowestRSI = DBL_MAX; for (int i = StochRSI_Period - 1; i >= 0; i--) { double currentRSI = iRSI(Symbol(), 0, StochRSI_Period, PRICE_CLOSE, i); if (currentRSI > highestRSI) highestRSI = currentRSI; if (currentRSI < lowestRSI) lowestRSI = currentRSI; } // Calculate Stochastic Oscillator for RSI double stochRSI = 0.0; if (highestRSI != lowestRSI) { stochRSI = (rsiValue - lowestRSI) / (highestRSI - lowestRSI) * 100; } // Assign the value to StochRSIValue StochRSIValue = stochRSI; // Calculate StochRSI %D (using StochRSI_DPeriod) int periodForD = StochRSI_DPeriod; double sumForD = 0.0; for (int i = 0; i < periodForD; i++) { sumForD += StochRSIValue; } double StochRSID = sumForD / periodForD; // Now, I have both %K (StochRSIValue) and %D (StochRSID) values for StochRSI } else { Print("Invalid input parameters for StochRSI calculation."); }
I am baffled to the method you suggested earlier. I'd be happy to hear what method you have..
Are you using chatgpt?
You need an array to memorize previous values of stochRSI to calculate the %K.
Also You need an array to memorize previous values of %K to calculate the %D.Are you using chatgpt?
You need an array to memorize previous values of stochRSI to calculate the %K.
Also You need an array to memorize previous values of %K to calculate the %D.For the implemetation of getting ideas then changing it to my design and state yes that is it
the other thing is when i add arrays the expert is throwing errors
That is if you have any other way to have them stored there without it breaking and throwing errors
If you are using ChatGPT and then coming here to request help with it, then that is not acceptable.
It produces horrible code, mixing MQL4 and MQL5, which cannot be easily fixed by newbie coders.
Either you know how to fix the code yourself, or please stop using ChatGPT.
No help will be provided for ChatGPT generated code on this forum.
If you are using ChatGPT and then coming here to request help with it, then that is not acceptable.
It produces horrible code, mixing MQL4 and MQL5, which cannot be easily fixed by newbie coders.
Either you know how to fix the code yourself, or please stop using ChatGPT.
No help will be provided for ChatGPT generated code on this forum.
I see. No Harm intended though. good to know this detail.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
My existing MQL4 code relies on the following external parameters, which users can customize according to their preferences:
I highly appreciate the assistance of the MQL4 community in helping to modify my existing code to create the Stochastic RSI indicator as described above. Any guidance, code examples, or insights would be greatly appreciated. If you need further details or code snippets, please let me know.
for now all i see in strategy tester in mt4 is the rsi indicator. The second image is what i'm trying to achieve.
Thank you in advance for your help!
Regards,
Benjamin