Hello
i am trying to code an inner bars indicator , (bars not 1 or 2 bars)
scan the last 10 bars and pick the main bar that its high and low did not break by body of any bars after it .
the logic to code this is getting out of mind completely .
i appreciate any direction , thanks
Hello
The high and low or the high from open , close and the low from open ,close ?
The scan starts 10 bars back or you want it to be unbroken for 10 bars to its right ?
Hello
The high and low or the high from open , close and the low from open ,close ?
The scan starts 10 bars back or you want it to be unbroken for 10 bars to its right ?
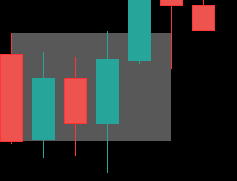
as an example , the hi /low if the first candle was not broken untill candle index3 and broke it with body . breaking with wicks are not counted .
so its just hi/low not high from open
the scan just for 10 last candle as more than that is not needed , not the inside bars , the inside bars could range from 1 till 10 .
hope its clear
as an example , the hi /low if the first candle was not broken untill candle index3 and broke it with body . breaking with wicks are not counted .
so its just hi/low not high from open
the scan just for 10 last candle as more than that is not needed , not the inside bars , the inside bars could range from 1 till 10 .
hope its clear
Try this :
#property copyright "Go to the discussion" #property link "https://www.mql5.com/en/forum/450585" #property version "1.00" #property indicator_chart_window #define SYSTEM_TAG "IBR_" #property indicator_buffers 5 #property indicator_plots 2 //--- plot IBTop #property indicator_label1 "Up" #property indicator_type1 DRAW_ARROW #property indicator_color1 clrRoyalBlue #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- plot IBBottom #property indicator_label2 "Down" #property indicator_type2 DRAW_ARROW #property indicator_color2 clrCrimson #property indicator_style2 STYLE_SOLID #property indicator_width2 1 input int ScanLimit=300;//Initial bars to scan (for testing) input int ScanRange=10;//Scan range for each IB (to its right) input int MinForSignal=3;//Min Length for signal input color Fill=clrRed;//Color //--- indicator buffers double IBUp[],IBDown[]; double IBTopBuffer[]; double IBBottomBuffer[]; double IBValid[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping ObjectsDeleteAll(ChartID(),SYSTEM_TAG); IBRCO=0; SetIndexBuffer(0,IBUp,INDICATOR_DATA); PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0); PlotIndexSetInteger(0,PLOT_ARROW,233); SetIndexBuffer(1,IBDown,INDICATOR_DATA); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0); PlotIndexSetInteger(1,PLOT_ARROW,234); SetIndexBuffer(2,IBTopBuffer,INDICATOR_DATA); SetIndexBuffer(3,IBBottomBuffer,INDICATOR_DATA); SetIndexBuffer(4,IBValid,INDICATOR_DATA); reset(); //--- return(INIT_SUCCEEDED); } void reset(){ ArrayInitialize(IBUp,0.0); ArrayInitialize(IBDown,0.0); ArrayInitialize(IBTopBuffer,0.0); ArrayInitialize(IBBottomBuffer,0.0); ArrayInitialize(IBValid,0.0); } void OnDeinit(const int reason){reset();ObjectsDeleteAll(ChartID(),SYSTEM_TAG);IBRCO=0;} //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int from=rates_total-1; int to=rates_total-2; if(prev_calculated==0){ from=rates_total-1-ScanLimit; if(from<1){from=1;} } else if(prev_calculated==rates_total){ from=rates_total; } else if((rates_total-prev_calculated)==1){ from=rates_total-2; } for(int i=from;i<=to;i++){ //if we in a valid extend it if(IBValid[i-1]>0.0) { IBValid[i]=IBValid[i-1]+1.0; //propagate IBTopBuffer[i]=IBTopBuffer[i-1]; IBBottomBuffer[i]=IBBottomBuffer[i-1]; if(((int)MathFloor(IBValid[i]))>MinForSignal){ EditIBR(IBRCO,time[i]); } }else{ IBValid[i]=0.0; IBTopBuffer[i]=0.0; IBBottomBuffer[i]=0.0; IBUp[i]=0.0; IBDown[i]=0.0; } //if IBValid above the limit kill it if(((int)MathFloor(IBValid[i]))>ScanRange){killIt(i);} //if in IBValid and the body slams it , kill it if(((int)MathFloor(IBValid[i]))>=MinForSignal){ if(close[i]>IBTopBuffer[i]){ IBUp[i]=close[i]; killIt(i); } else if(close[i]<IBBottomBuffer[i]){ IBDown[i]=close[i]; killIt(i); } } //if not valid start it if(IBValid[i]==0.0){ IBTopBuffer[i]=high[i]; IBBottomBuffer[i]=low[i]; IBValid[i]=1.0; } //if 2 section draw if(((int)MathFloor(IBValid[i]))==MinForSignal){ createIBR(time[i-(MinForSignal-1)],time[i],IBTopBuffer[i],IBBottomBuffer[i],Fill); } } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ void killIt(int i){ IBValid[i]=0.0; IBTopBuffer[i]=0.0; IBBottomBuffer[i]=0.0; } int IBRCO=0; void createIBR(datetime time_from, datetime time_to, double top, double bottom, color col){ IBRCO++; string name=SYSTEM_TAG+"_"+IntegerToString(IBRCO); ObjectCreate(ChartID(),name,OBJ_RECTANGLE,0,time_from,top,time_to,bottom); ObjectSetInteger(ChartID(),name,OBJPROP_BACK,true); ObjectSetInteger(ChartID(),name,OBJPROP_COLOR,col); ObjectSetInteger(ChartID(),name,OBJPROP_FILL,true); ChartRedraw(0); } void EditIBR(int co,datetime new_time_to){ string name=SYSTEM_TAG+"_"+IntegerToString(IBRCO); ObjectSetInteger(ChartID(),name,OBJPROP_TIME,1,new_time_to); ChartRedraw(0); }
Lorentzos , cant thank you enough, Great work, Thanks a lot !!
just one thing , the color of the rectangle object is not changing , weird
in line 24 i changed it in code but it still getting back to red (not crimson) color
Lorentzos , cant thank you enough, Great work, Thanks a lot !!
just one thing , the color of the rectangle object is not changing , weird
in line 24 i changed it in code but it still getting back to red (not crimson) color
You are welcome!
Yeah my bad , the FILL parameter means (fill true/false)
i will update the code

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello
i am trying to code an inner bars indicator , (bars not 1 or 2 bars)
scan the last 10 bars and pick the main bar that its high and low did not break by body of any bars after it .
the logic to code this is getting out of mind completely .
i appreciate any direction , thanks