I have this simple code above.
For some reason the stop loss and take profit from is not triggering as the .png shows.
Can anyone help me see what i have done wrong?
Thanks in advance!
Buy at Ask
Sell at Bid
Why use Open Price?
Thanks for replying me!
I'm newbie so i'm learning yet.
Well, the strategy i want to do requires the order send with the open price right after a specif candle.
I thought that i could send any price to trade.Buy with the SL and TP i have set according to the open price.
if it's not too much trouble, could you give me an example or provide a link to it?
I put an update section on my first post with a try to do what you told.
Again, thank you very much!
In might be best for you to place your question in the Portuguese forum.
You are dealing with a symbol and exchange that has a special and peculiar behaviour which is unique to Brazil.
Most of the traders here don't have any experience with Brazilian markets or those unique conditions.
Brazilian traders/codes will be able to offer better advice based on their experiences on the same market.
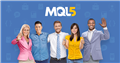
- www.mql5.com
I am not sure which assets you are working with but let’s assume it is currency pairs
your takeprofit is 5 and current price is 1.0015
which means you want trade to close when price is = 5+1.0015 = 6.0015, Not feasible…
Use this instead
if(candleG == 5){ double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol, SYMBOL_ASK), _Digits); double Bid = NormalizeDouble(SymbolInfoDouble(_Symbol, SYMBOL_BID), _Digits); double tp = Ask + takeprofit*_Point; //NOTE I used Ask for Buys and Bid for Sells double sl = Ask - stoploss*_Point; bool ticket = trade.Buy(1, _Symbol, Ask, sl, tp, "[BUY]"); if(ticket > 0){ Print("Success! Ticket: ", trade.ResultDeal()); } else { Print("Error code: ", GetLastError()); } }
Wrong, that is not what a limit order does. It means that price or better.
double tp = Ask + takeprofit*_Point; //NOTE I used Ask for Buys and Bid for Sells double sl = Ask - stoploss*_Point;
-
Floating point has an infinite number of decimals, it's you were not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum #10 (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
-
Floating point has an infinite number of decimals, it's you were not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum #10 (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have this simple code above.
For some reason the stop loss and take profit from is not triggering as the .png shows.
Can anyone help me see what i have done wrong?
Thanks in advance!
----------------------- UPDATE ---------------------------------
I tried the code below but still get the same situation