Please EDIT your post and use the CODE button when you insert code.
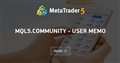
- www.mql5.com
Why arte you trying what already exist: https://www.mql5.com/en/search#!keyword=ma%20crossing%20EA&module=mql5_module_codebase
Learn to search is faster then learning to code
Please don't post randomly in any section. Your question is not related to the section you posted.
MT4/mql4 has it's own section on the forum. I have moved your topic to the correct section.
Please don't post randomly in any section. Your question is not related to the section you posted.
MT4/mql4 has it's own section on the forum. I have moved your topic to the correct section.
Why arte you trying what already exist: https://www.mql5.com/en/search#!keyword=ma%20crossing%20EA&module=mql5_module_codebase
Learn to search is faster then learning to code
Thanks Carl for the assist.
I was just trying to learn from some basics.
But still is it possible for you to check and let me know my fault in saving the ticket number and closing it. It would be of great help.
-
int ticket = OrderSend(NULL,OP_BUY,lot_size,Ask,slippage,0,0,"Buy",0,0,clrGreen);
Be careful with NULL.
- On MT4, you can use NULL in place of _Symbol only in those calls that the documentation specially says you can. iHigh does, iCustom does, MarketInfo does not, OrderSend does not.
- Don't use NULL (except for pointers where you explicitly check for it.) Use _Symbol and _Period, that is minimalist as possible and more efficient.
- Zero is the same as PERIOD_CURRENT which means _Period. Don't hard code numbers.
- MT4: No need for a function call with iHigh(NULL,0,s) just use the predefined arrays, i.e. High[].
MT5: create them. - Cloud Protector Bug? - MQL4 programming forum (2020)
-
if(OrdersTotal()==0)
Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number/symbol filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum (2013)
PositionClose is not working - MQL5 programming forum (2020)
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles (2006)
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles (2011)
Limit one open buy/sell position at a time - General - MQL5 programming forum (2022)You need one Magic Number for each symbol/timeframe/strategy.
Trade current timeframe, one strategy, and filter by symbol requires one MN.
If trading multiple timeframes, and filter by symbol requires use a range of MN (base plus timeframe).
Why are MT5 ENUM_TIMEFRAMES strange? - General - MQL5 programming forum - Page 2 #11 (2020)
Hi, i am very new to mql. I was trying to code a basic ma crossover, but i could open the order but not close it. Can someone help me where i am going wrong.
I am a novice but I am mostly sure that you need to OrderSelect() aka (Select) the order before you can OrderClose() or Modify it.
https://docs.mql4.com/trading/orderticket
if(ticket>0)
{
OrderSelect(ticket,SELECT_BY_TICKET);
if(signal ==1 && lastclose_price>lastma && close_price<ma)
OrderClose(ticket, lot_size, Bid, slippage, clrNONE);
else if(signal ==-1 && lastclose_price<lastma && close_price>ma)
OrderClose(ticket, lot_size, Ask, slippage, clrNONE);
}
}
There is many other methods you will discover but you will also want to learn how to GetLastError().
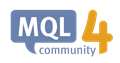
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, i am very new to mql. I was trying to code a basic ma crossover, but i could open the order but not close it. Can someone help me where i am going wrong.