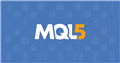
- www.mql5.com
- MQL5 Ordersend for Binary Options?
- Object Oriented Approach to MQL
- Help me fix this code pls
As per Forum rules and recommendations, please edit your post (don't create a new post) and replace your code properly (with "</>" or Alt-S).
NB! Very important! DO NOT create a new post. EDIT your original post.
Do you know how to code?
This seems like ChatGPT generated code, mixing MQL4 and MQL5.
Please stop using ChatGPT. It is horrendous.
Please dedicate some time and effort into learning MQL properly, or hire a human coder.
Your code | double highPrice = 0; double lowPrice = 0; for(int i = 0; i < 7; i++) { if(i == 0) { highPrice = rates[i].high; lowPrice = rates[i].low; } else { if(rates[i].high > highPrice) highPrice = rates[i].high; if(rates[i].low < lowPrice) lowPrice = rates[i].low; } } |
Simplified | double highPrice = rates[0].high; double lowPrice = rates[0].low; for(int i = 1; i < 7; i++) { if(rates[i].high > highPrice) highPrice = rates[i].high; if(rates[i].low < lowPrice) lowPrice = rates[i].low; } |
How do you want us to help, if you don't understand coding?
First, you a missing a closing brace "}" at the very end.
Second, after you fix that, you will have 7 new errors due to mixing MQL4 and MQL5 code, where "AccountBalance()" and "OrderOpenPrice()" are MQL4 code and not MQL5.
You also have some outdated MQL5 code practices as well.
Was that understandable for you?
How do you want us to help, if you don't understand coding.
First, you a missing a closing brace at the very end.
Second, after you fix that, you will have 7 new errors due to mixing MQL4 and MQL5 code, where "AccountBalance()" and "OrderOpenPrice()" are MQL4 code and not MQL5.
You also have some outdated MQL5 code practices as well.
Actually, it was very hard. It took a very long time (years) and plenty of effort to learn the two MQL languages and to master them both—a lot of dedication to gain that knowledge and skill to be able to answer you.
Actually, it was very hard. It took a very long time (years) and plenty of effort to learn the two MQL languages and to master them both—a lot of dedication to gain that knowledge and skill to be able to answer you.
-
Is it easy (or difficult) to …
Actually, it was very hard. It took a very long time (years) and plenty of effort to learn the two MQL languages and to master them both—a lot of dedication to gain that knowledge and skill to be able to answer you.
I have no doubt in my mind that learning those 2 languages was extremely hard(that is not a sarcasm i rly think that), but that wasn't my point when i wrote my comment. But i will end the disquction here since i kinda got it to work, its a bootstrap and in theory it should work, its just not fully automated as i intended :D

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use